Add Point/Annotation to Map View With Swift
Learn how to easily add MKPointAnnotation's to your maps so that you can give more context to a location
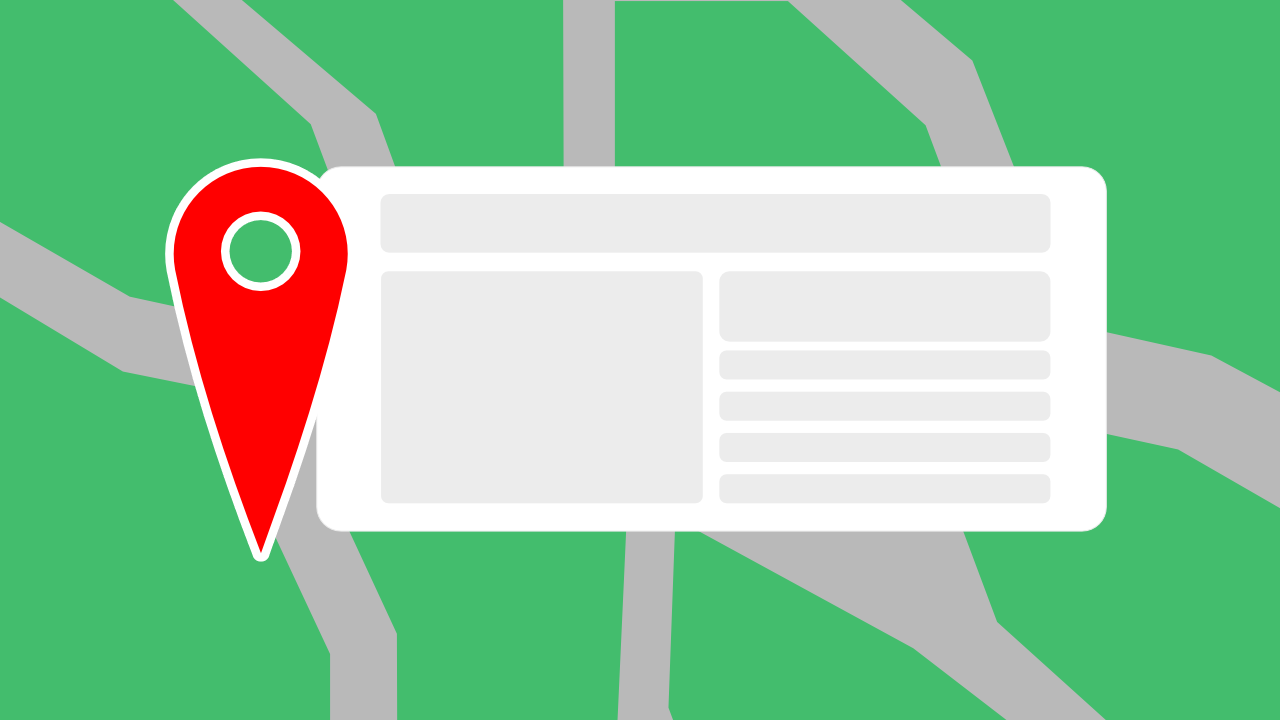
Adding a MKPointAnnotation on a map with Swift is quite simple and in this tutorial I will show you how to add a annotaiton to a MKMapView with Swift.
For this tutorial I will assume that you have already setup your map view. If you haven’t I have another tutorial, Getting started with MKMapView, that will help you to get started with MKMapView.
Ok, lets get started.
Step 1: Creating the annotation
// Create MKPointaAnnotation
let annotation = MKPointAnnotation()
annotation.title = "London"
annotation.coordinate = CLLocationCoordinate2D(latitude: CLLocationDegrees(51.5074),
longitude: CLLocationDegrees(0.1278))
The above code is pretty self explanatory. We create a new instance of MKPointAnnotation, after that we set the title property to London
and then we create a new instance of CLLocationCoordinate2D
with the latitude and longitude of London
.
Step 2: Adding a MKPointAnnotation to an MKMapView
This is even easier than the previous step. The map view has a method called addAnnotation
, we will use this to add the annotation we have created.
// Add annotation to mapView
self.mapView.addAnnotation(annotation)
And you are done! If you want to add multiple MKPointAnnotations’ there is another method called addAnnotations
. This will take an array of MKPointAnnotations’.
In the below example just assume that I have created two instances of MKPointAnnotations called londonAnnotation
and newYorkCityAnnotation
, in the same way that we created it before.
// Add multiple annotations.
let annotations = [londonAnnotation, newYorkCityAnnotation]
self.mapView.addAnnotations(annotations)
That is how simple it is to add single or multiple annotations to a MKMapView.