Add UIButton programmatically using Swift
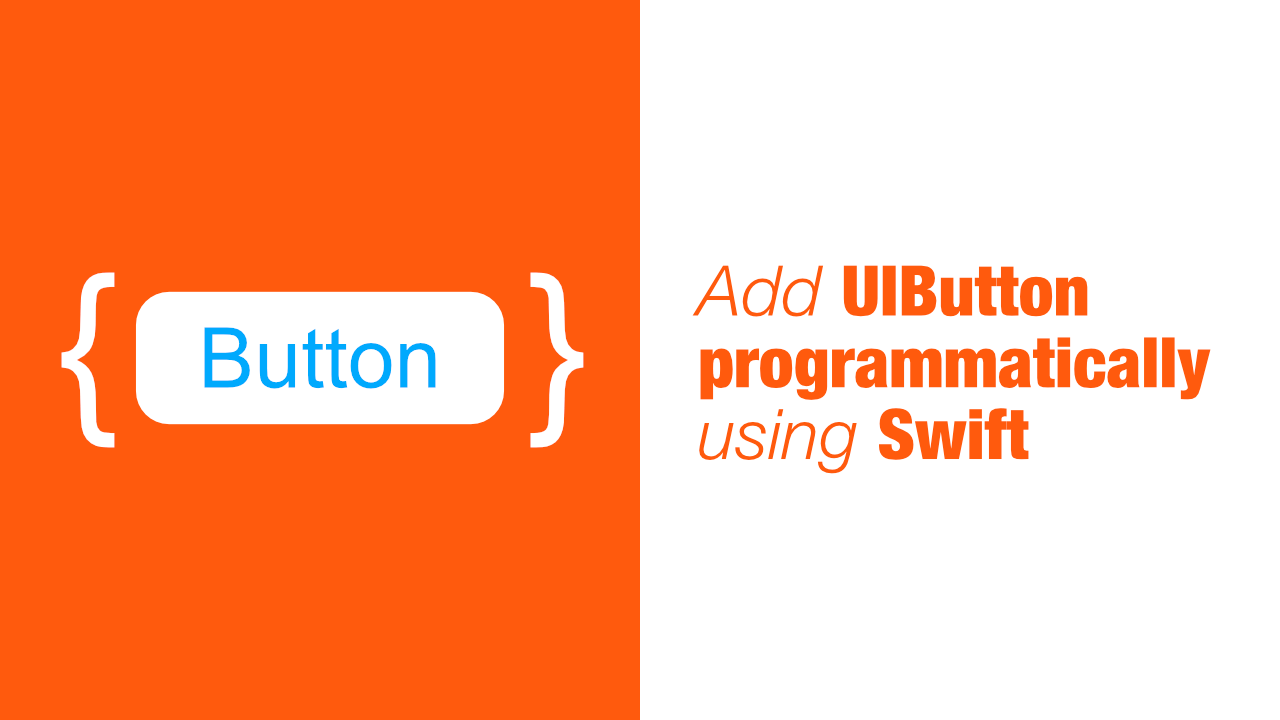
There could be situations where one needs to create a button programmatically. In this tutorial we will learn how to add a button using code.
Create the button action
Before we start with the button we need to start with create the selector that we will use for the button action.
All this selector will do is print out Button pressed
so let's add the following to our code:
@objc
func buttonAction() {
print("Button pressed")
}
Create the button
I will be creating this button in my viewDidLoad
, but you can add it wherever works best for you.
In the following code we will be initialising a new UIButton
. Add the following to your code:
let button = UIButton(frame: CGRect(x: 100,
y: 100,
width: 200,
height: 60))
Set button title
Next we will set our button title, add the following to your code:
button.setTitle("Test",
for: .normal)
Set button title color
The next thing that we need to do is to set our button title's color. The following code will let us do that:
button.setTitleColor(.systemBlue,
for: .normal)
Set the button action
Setting the button action programmatically is simple, and can also be used if your button has been created using Interface Builder.
To add the action to our button we need to add the following code:
button.addTarget(self,
action: #selector(buttonAction),
for: .touchUpInside)
Add button as a subview
The last thing that we need to do is to add this button as a subview. To do that, add the following to your code:
self.view.addSubview(button)
If we build and run the code we should see the following:
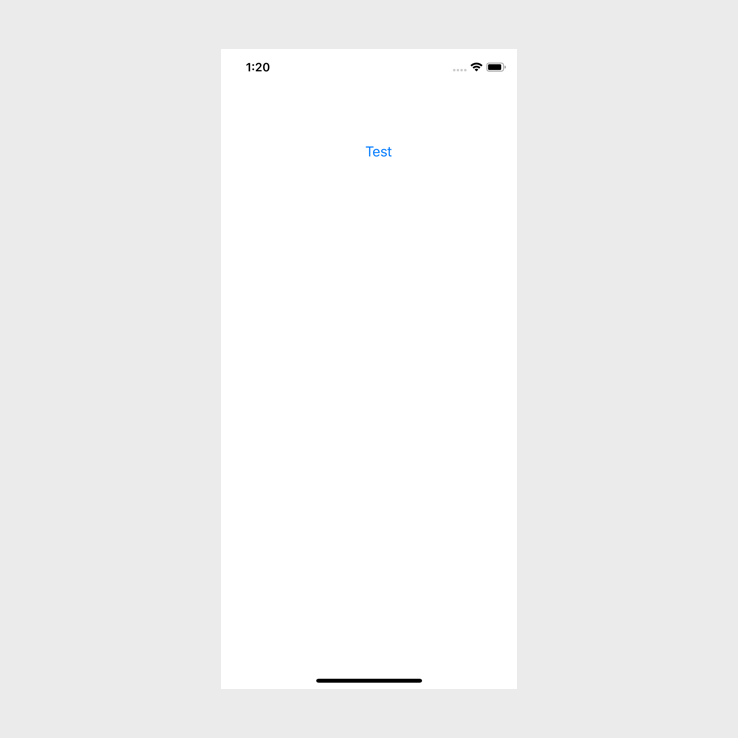
And if we tap on the button, Button pressed
should be printed in our console in Xcode.
This is the full source code for the view controller:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let button = UIButton(frame: CGRect(x: 100,
y: 100,
width: 200,
height: 60))
button.setTitle("Test",
for: .normal)
button.setTitleColor(.systemBlue,
for: .normal)
button.addTarget(self,
action: #selector(buttonAction),
for: .touchUpInside)
self.view.addSubview(button)
// Do any additional setup after loading the view.
}
@objc
func buttonAction() {
print("Button pressed")
}
}