Change button color on press with Flutter
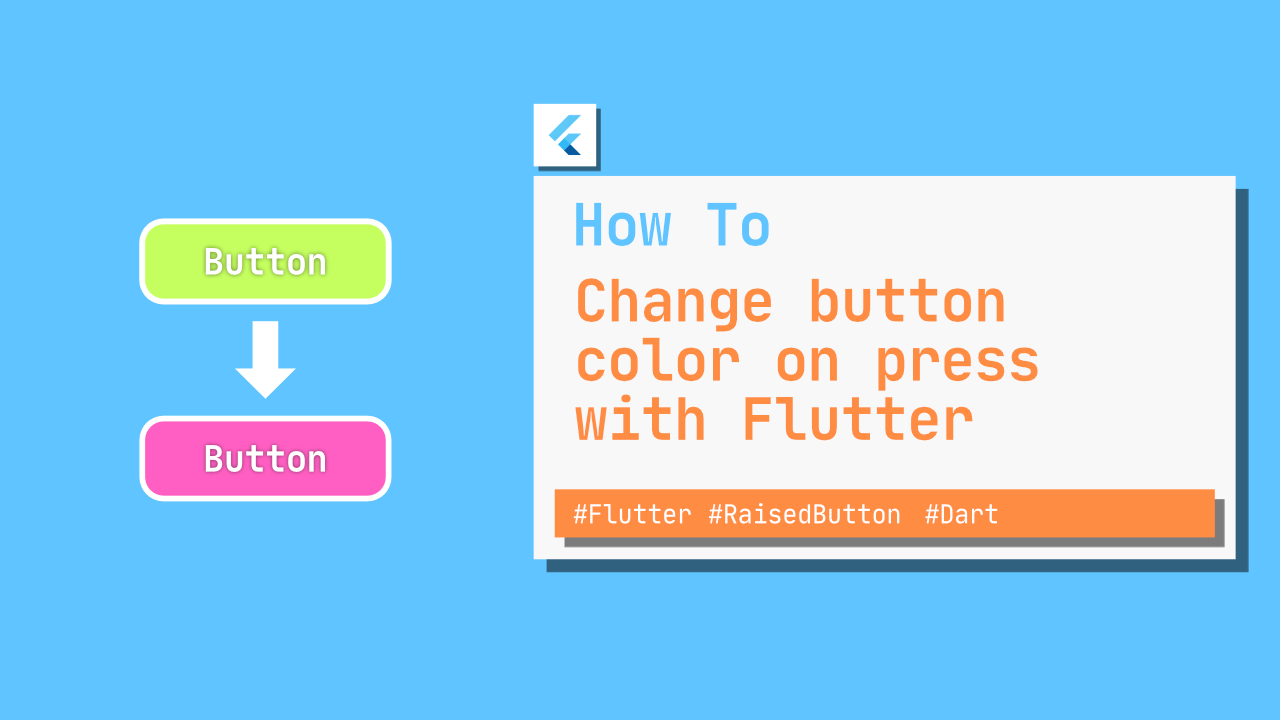
In this tutorial I will show you how you can change the color of a button when a user presses it. To demonstrate this I will be using a RaisedButton
.
Toggle button color change on press
class _MyHomePageState extends State<MyHomePage> {
// 1
bool _hasBeenPressed = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
RaisedButton(
child: new Text('Change Color'),
textColor: Colors.white,
// 2
color: _hasBeenPressed ? Colors.blue : Colors.black,
// 3
onPressed: () => {
setState(() {
_hasBeenPressed = !_hasBeenPressed;
})
},
)
],
),
),
);
}
}
In the above code there are a few things to look out for:
- I have a field called
_hasBeenPressed
. This field is abool
and we will use this to toggle thecolor
of theRaisedButton
. - The
color
property that we want to change. We will use a ternary operator which will switch between blue or black depending on the current state of the_hasBeenPressed
field. - Lastly, we use
onPressed
to toggle and set the state.
Cycle through button color on press
This will be based off of the above code, but we will be changing parts 1, 2 and 3.
Let's replace _hasBeenPressed
field with the following:
List<Color> _colors = <Color>[
Colors.blue,
Colors.red,
Colors.black,
Colors.green
];
int _currentColorIndex = 0;
void _incrementColorIndex() {
setState(() {
if (_currentColorIndex < _colors.length - 1) {
_currentColorIndex++;
} else {
_currentColorIndex = 0;
}
});
}
We are doing three things in the above code. The first is that we are create a simple List
that has four colors, these are the colors that we are going to cycle through.
Next, we have a field called _currentColorIndex
. This is the index for the current color that we will be using.
The last part of the above code is _incrementColorIndex
. In this function we will increment _currentColorIndex
by one. If the _currentColorIndex
value is smaller than the number of colors that we have. If it is not smaller we will set it to 0
.
Update color property
Now that we have all the logic and setting of state out of the way, let's update the color
property for RaisedButton
.
Previously we used a ternary operator to decide what the buttons color would be, but now we can replace the ternary operator with the following:
_colors[_currentColorIndex],
Update onPressed
Lastly we need to update onPressed
. Previously we were setting the state directly from onPressed
but now we will call _incrementColorIndex
. Update onPressed
to look like this:
() => {_incrementColorIndex()}
Conclusion
And that is it. That is how easy it is to change the button color with Flutter, whether you want to toggle between two colors or cycle between a few colors, all we need to do is change the color
property for the button.
If you want the full source code for this, you can find it here.