Change UITableView separator color
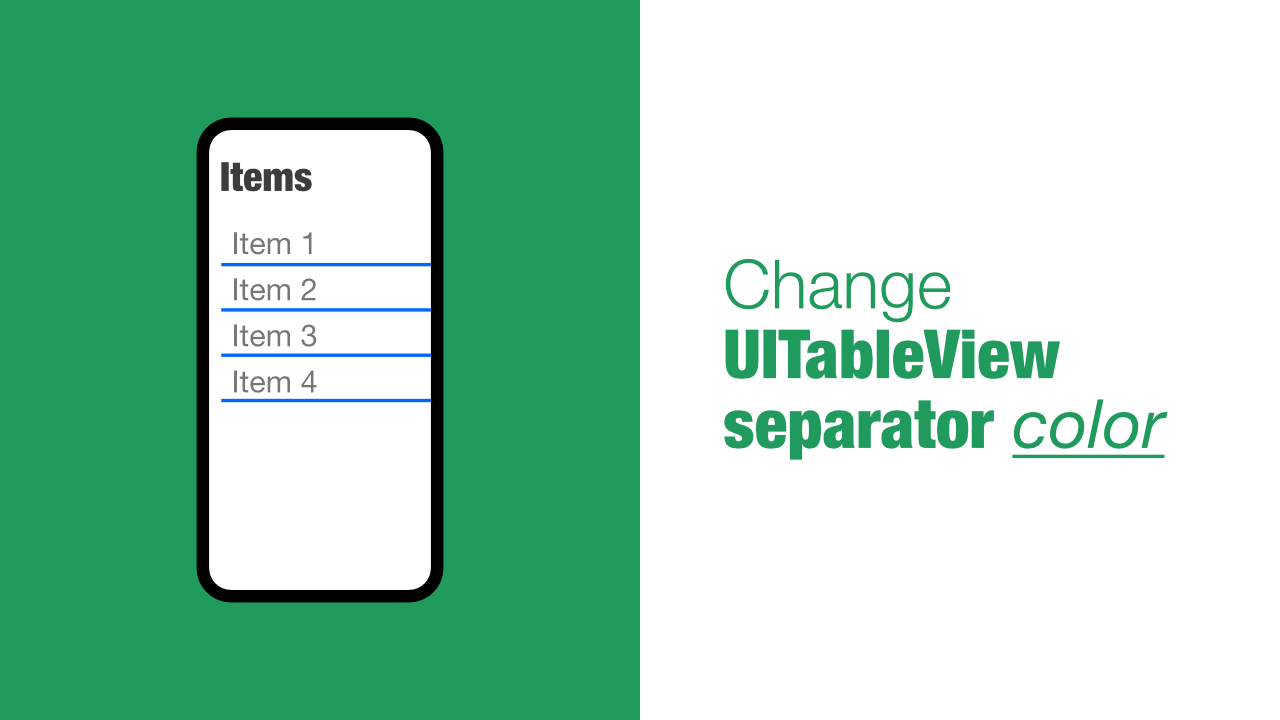
In this quick tutorial I will show you how to change a UITableView's separator color. There are two ways to do this, both are very easy, but there is one that I personally prefer to use.
Links
- Change separator color with Interface Builder
- Change separator color with Swift(my preferred method)
Change UITableView separator color with Interface Builder
Before we get into using Interface Builder
, this is what the app currently looks like:
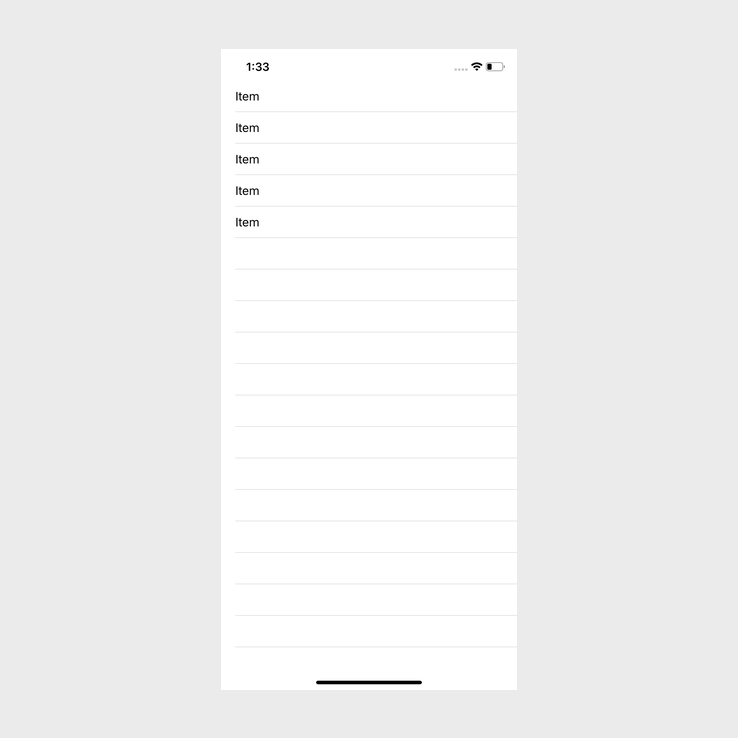
To change the separator color using Interface Builder
we need to open the storyboard/xib file. Once storyboard/xib file is open, select the UITableView that you want to change the separator color for.
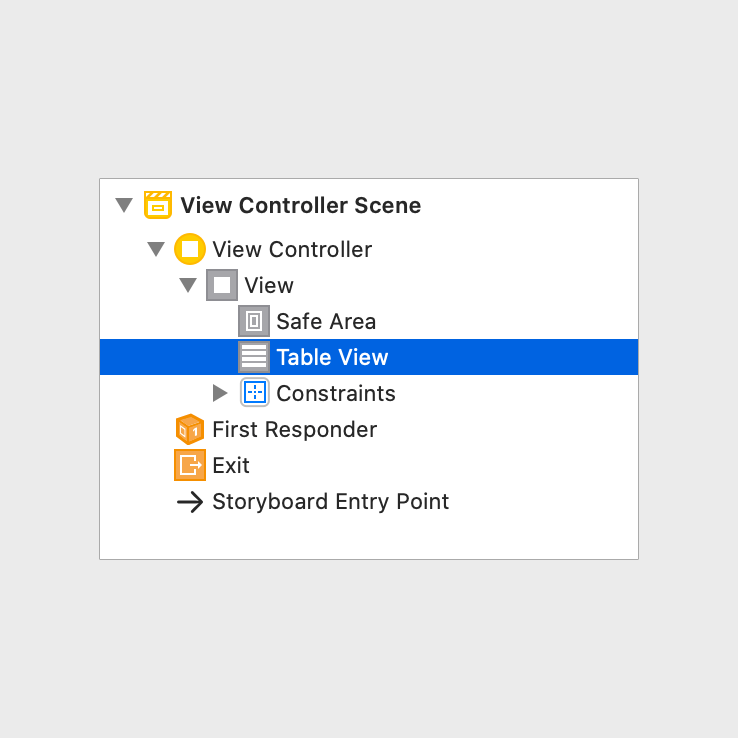
Now that we have selected the UITableView, we need to go to the Attribute Inspector
. Under Separator
you will see a drop down like as seen in the image below:
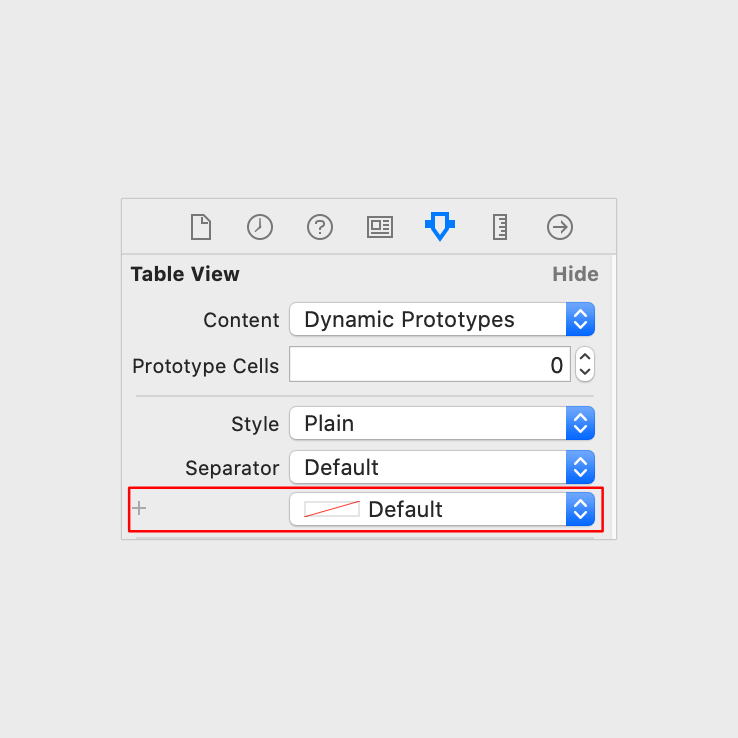
If you click on the drop down, you will see the following:
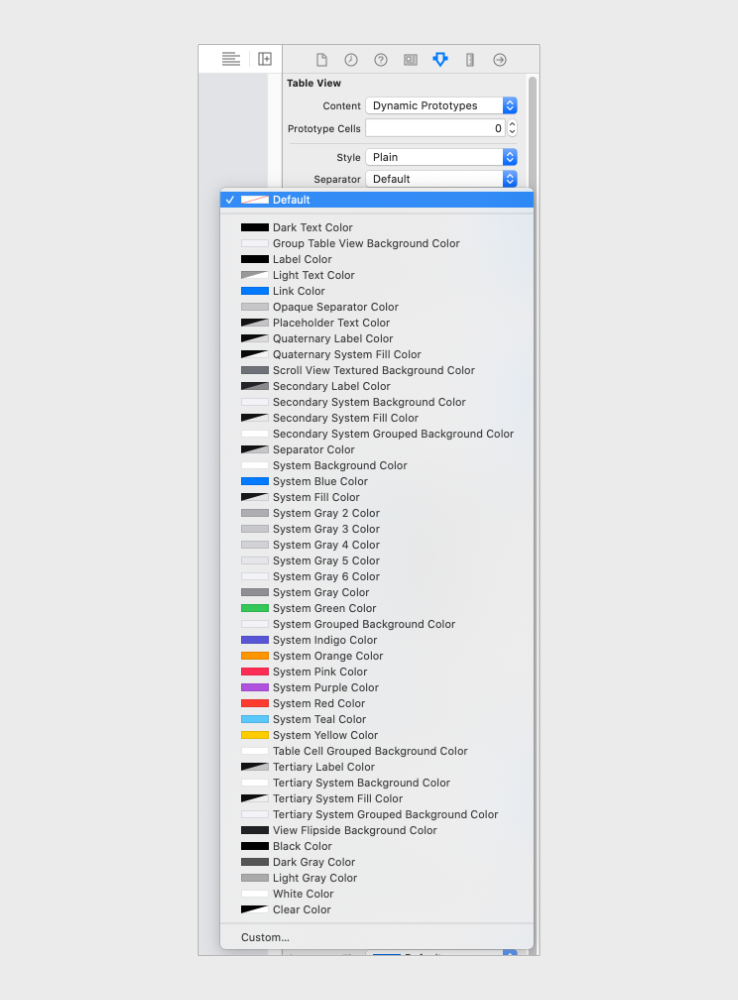
This will allow you to pick a color from the list provided, or select a custom color from the bottom of the list.
I have selected System Purple Color
, when I build and run my app, it looks like this:
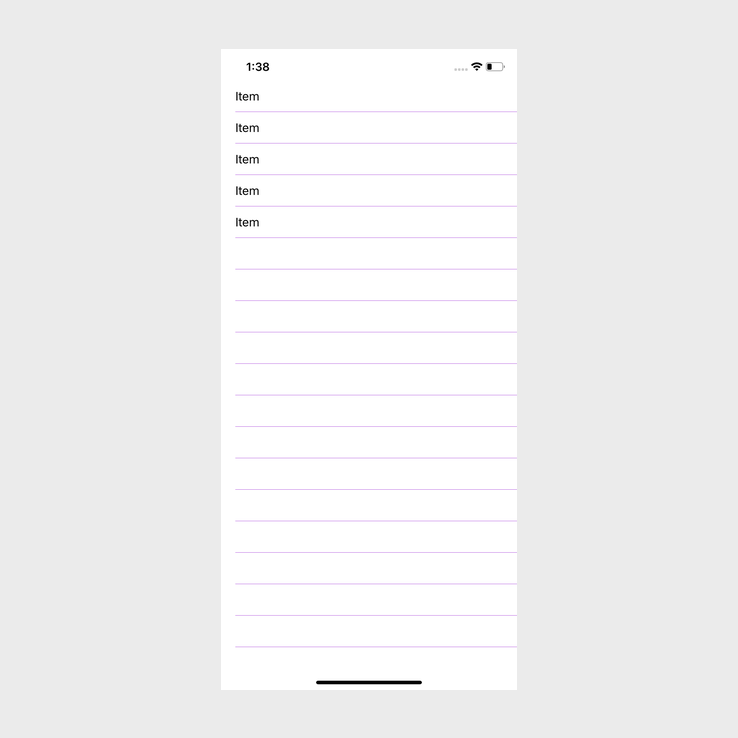
Change UITableView separator color with Swift
Using the Interface Builder
is extremely easy, but I personally prefer to have styling in code, and when it comes to changing the separator color, it is even easier to use code compared to using the Interface Builder
.
Let's take a look the ViewController
that I am using:
class ViewController: UIViewController, UITableViewDataSource {
let tableViewData = Array(repeating: "Item", count: 5)
@IBOutlet weak var tableView: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
tableView.register(UITableViewCell.self,
forCellReuseIdentifier: "TableViewCell")
tableView.dataSource = self
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.tableViewData.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "TableViewCell",
for: indexPath)
cell.textLabel?.text = self.tableViewData[indexPath.row]
return cell
}
}
This code contains the basic functionality of a UITableView. If I build and run the app, it will look like this:
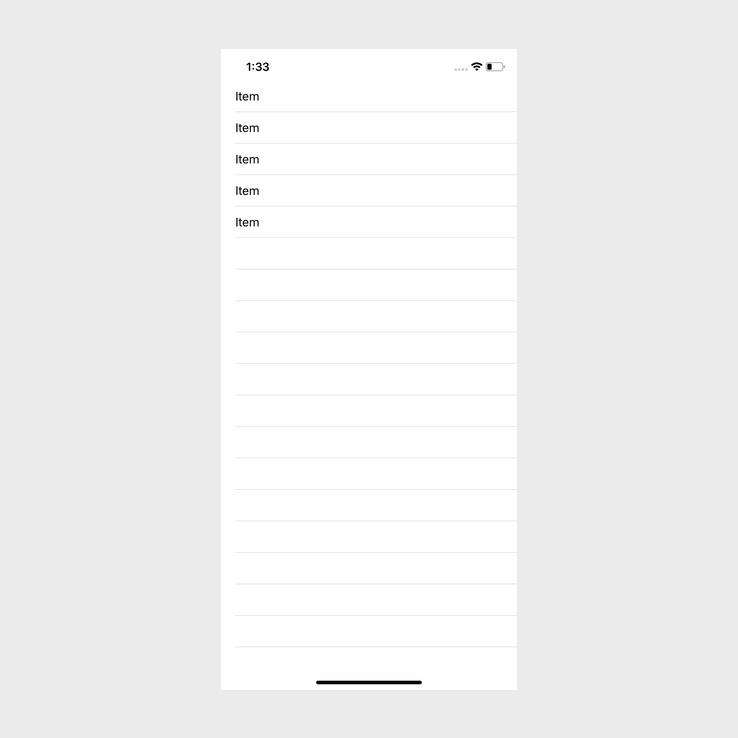
All that we need to do in order to change the color of the separator is to add the following line of code just below tableView.dataSource = self
in the viewDidLoad
method:
tableView.separatorColor = UIColor.red
After adding the above code to the end of viewDidLoad
, it should look like this:
override func viewDidLoad() {
super.viewDidLoad()
tableView.register(UITableViewCell.self,
forCellReuseIdentifier: "TableViewCell")
tableView.dataSource = self
tableView.separatorColor = UIColor.red
}
If I build and run the app now, it looks like this:
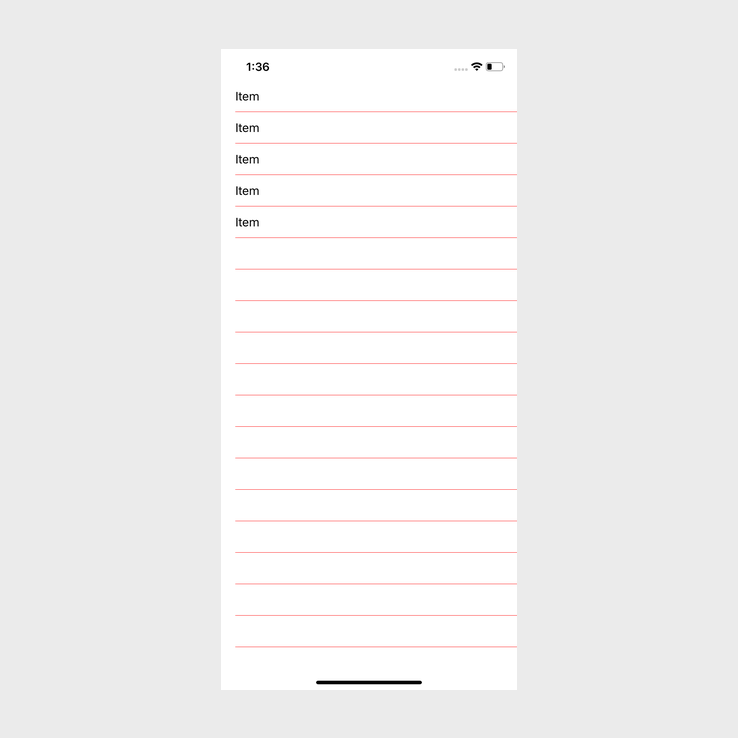
Conclusion
Changing the separator color on a UITableView is very easy no matter which way you do it. Personally I prefer doing it in code because one can easily make it reusable.
You can find the full source code here.