Change UITextField placeholder text color with Swift
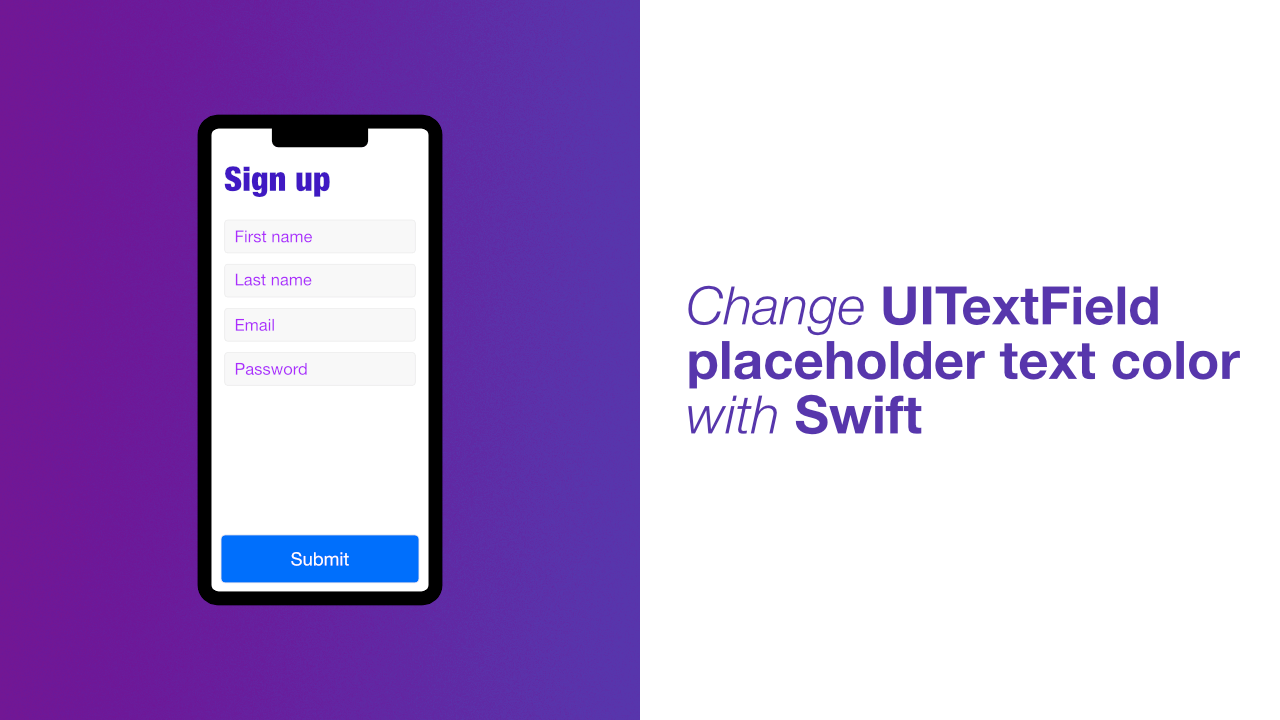
In this tutorial you will learn how to change the color of a UITextField's placeholder text. This is a straight forward task but if you are anything like me and you see NSAttributedString
you start looking for a different solution. We are going to be using NSAttributedString
in this tutorial, but it is very basic and easy for what we need to do.
Before we start, I am going to assume that you have a text field set up. If not, you will need a text field set up for this tutorial.
I have a text field outlet and I will be using the attributed string to change the color in the didSet
property observer. Update your text field outlet to look like this:
@IBOutlet weak var textField: UITextField! {
didSet {
let redPlaceholderText = NSAttributedString(string: "My Placeholder",
attributes: [NSAttributedString.Key.foregroundColor: UIColor.red])
textField.attributedPlaceholder = redPlaceholderText
}
}
As mentioned before, this is a very simple attributed string. All we have done is create a new variable and assigned a new instance of NSAttributedString
to it. The first argument of the attributed string is the string we want to display, and the second argument is for the attributes we want to use.
In the above example we are only using the .foregroundColor
attribute and setting it to UIColor.red
for simplicity. You could make this any color you want.
The last thing that we have done is used the attributedPlaceholder
property instead of the normal placeholder
property. This will allow us to assign an attributed string as the placeholder text.
If you do not have the text field as an outlet, you could create a function that will assign the attributed string to the text field as we have done in the code.
Conclusion
Using an attributed string is actually quite simple, even if it does seem daunting at first. There are quite a few options that an attributed string will allow you to customise, such as the font, stroke color, stroke width, kern etc.
If you want to see my full source code, you can find it here.