Difference Between Methods and Functions in Swift
In this tutorial we will learn what the difference is between a method and function in Swift
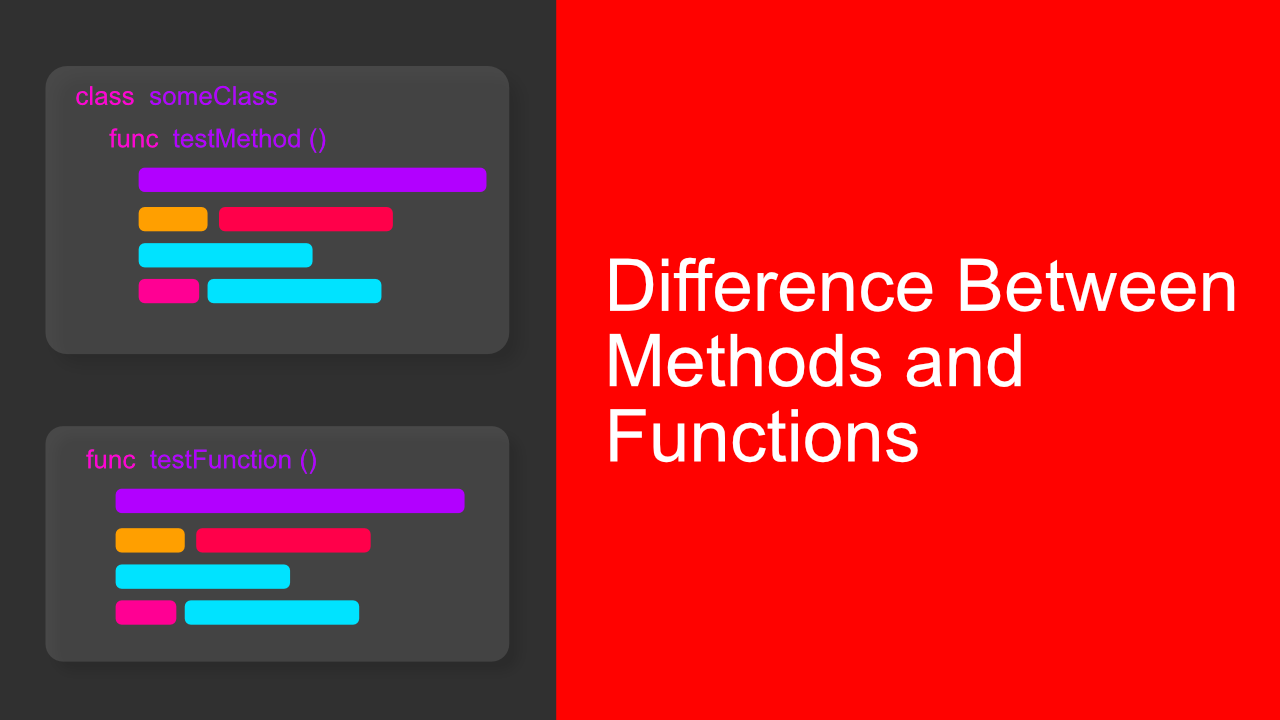
I never really thought that there was a difference between a method
and a function
, and this probably does not deserve its own post, but in this post I will explain the difference between a method
and a function
in Swift.
Functions
A function
is just some code that will do a specific task. These functions must not be associated with a particular type.
Basically these are global functions and they can be added to any file outside of a particular type.
Methods
A method
is a function
but it is associated with a particular type.
Two types of methods
There are two types of methods. Instance methods and type methods. An instance method is a function that will belong to a specific instance of a class, struct or enum. Instance methods add functionality to an instance and works within that instance.
Instance methods
For example. If you have class call Car
, you might also have an instance method called start
. This instance method will update a property on the car called running
.
class Car
{
var running: Bool = false
func start()
{
self.running = true
}
}
let car = Car()
car.start()
As you can see in the above code. We have a Car
class that has an instance property and an instance method. All this means is that one needs to create an instance of Car
to be able to use or access these properties or methods.
This is different to Type
methods which is explained below.
Type Methods
A type method is basically a static
method. It does not need an instance of the class to run. Below I take the same example as above with the Car
class, but I have added a static
method called ‘make’. All this type method will do is create a new instance of Car
.
I have also added a new static
property called numberOfInstances
. This will keep track of the number of instances we have created of type Car
.
The make
method will increment the numberOfInstances
property every time it gets called.
class Car
{
static numberOfInstances = 0
var running: Bool = false
func start()
{
self.running = true
}
static func make() -> Car {
Car.numberOfInstances += 1
return Car()
}
}
I have removed the initialization code that I had before because we are going to use the new make
method. As you will see below, we do not have to create a new instance of Car
in order to use the make
method.
let car = Car.make()
car.start()
In the above code we created a new instance of car, once we have the new instance we can call the instance method start
. As you can see from the above code, we did not need to create an instance to use the make
method, we just called it directly on the Car
type.
Because the make
method and the numberOfInstances
property are both type properties, we can use them without an instance. We have seen how to use a type method now lets see how to use a type property.
let newCar = Car.make()
print("Number of instances: ", Car.numberOfInstances)
In the above code we create a new instance of Car
by using the make
method. After we have done this we can use the numberOfInstances
property. We do not need to create a new instance of this property, instead all we have to do is Car.numberOfInstances
. When we print this out we it should print out Number of instances: 2
, since we called the make
method twice.