Hashvalue Deprecated as Protocol Requirement
Fix Hashvalue Deprecated as Protocol Requirement
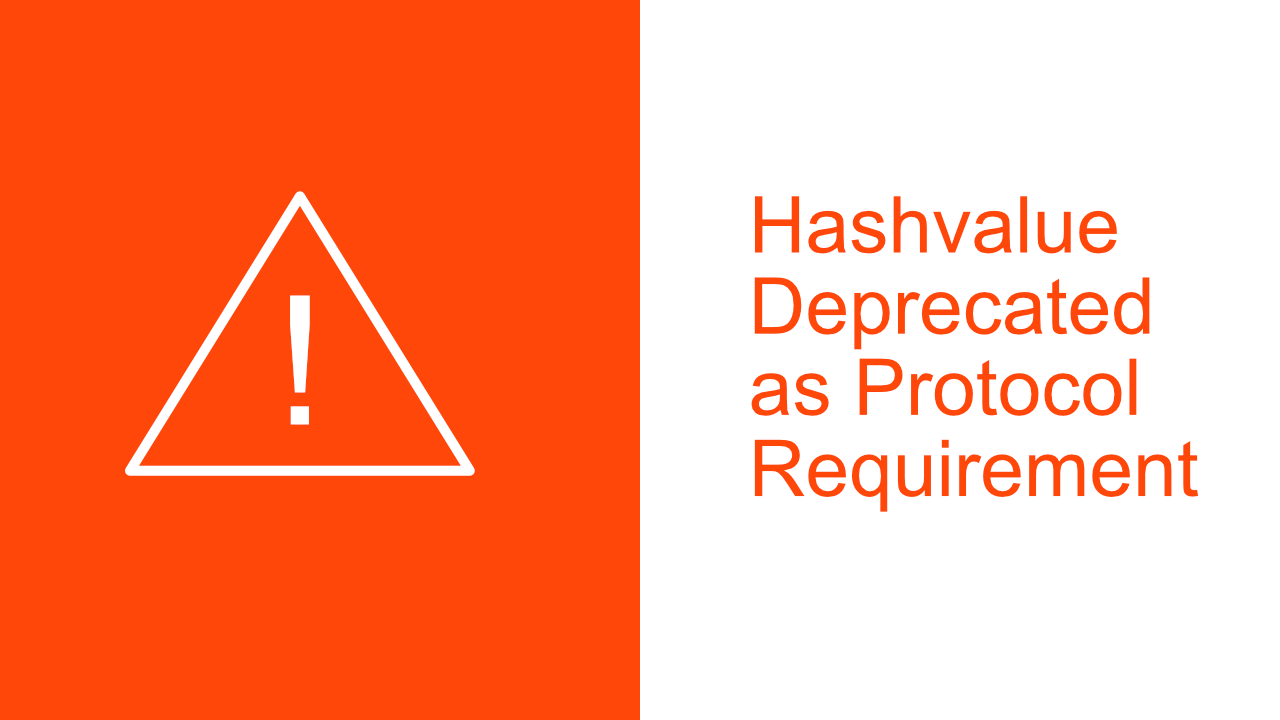
I have recently been going through warnings on a project I am working on. One of the warnings was 'Hashable.hashValue' is deprecated as a protocol requirement; conform type to 'Hashable' by implementing 'hash(into:)' instead
.
This confused me a bit so I decided to carry on with the other warnings. It turns out this is a really simple fix.
Here is an example of the issue:
enum ImageSize: Hashable {
case small
case medium
case large
public var hashValue: Int {
switch self {
case .small:
return 0
case .medium:
return 1
case .large:
return 2
}
}
}
The warning shows up when you are implementing the hashValue
. The fix for this is to remove the implementation for hashValue
and provide an implementation for the hash(into:)
function.
The updated code will look like this:
enum ImageSize: Hashable {
case small
case medium
case large
func hash(into hasher: inout Hasher) {
switch self {
case .small:
hasher.combine(0)
case .medium:
hasher.combine(1)
case .large:
hasher.combine(2)
}
}
}
Basically the fix is to take your return
and replace it with the hasher.combine
. The argument that the combine
method takes will be the same value that you were returning.
That will fix the warning because you are now correctly conforming to the Hashable
protocol.