How to add a delay to code in Swift
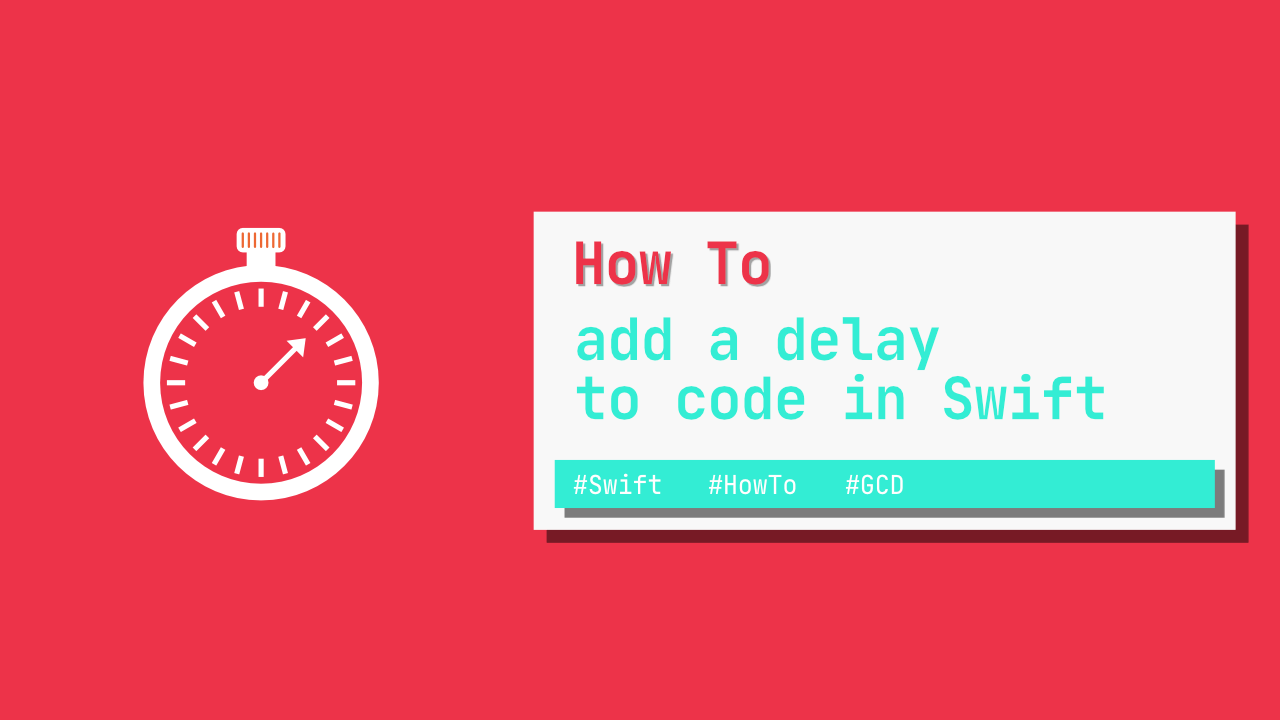
In this tutorial you will learn how to add a delay to your code. To add a delay to your code we need to use GCD
.
GCD
has a built in method called asyncAfter
, which will allow us to run code after a given amount of time.
The code is extremely simple:
print("Before delay")
DispatchQueue.main.asyncAfter(deadline: .now() + 2) {
print("Async after 2 seconds")
}
In the above code, Before delay
will be printed out first and after 2
seconds, Async after 2 seconds
will be printed.
There is a way to make the above code easier to read in my opinion. Instead of using 2
we can use .seconds(2)
. By default asyncAfter
uses seconds, so for the above example it might not be the best use case, however, if you wanted microseconds
or milliseconds
this will help.
Below is an example with milliseconds
:
print("Before delay")
DispatchQueue.main.asyncAfter(deadline: .now() + .milliseconds(500)) {
print("async after 500 milliseconds")
}
Conclusion
Adding a delay to code is easy with Swift but I wish that they would make it so that one wouldn't have to go through DispatchQueue.main
. Having said that, this is not something that should be used super often in most situations, and if you did need it often, you would just creation this function yourself.
You can find the full source code here.