How to compare dates with TypeScript or JavaScript
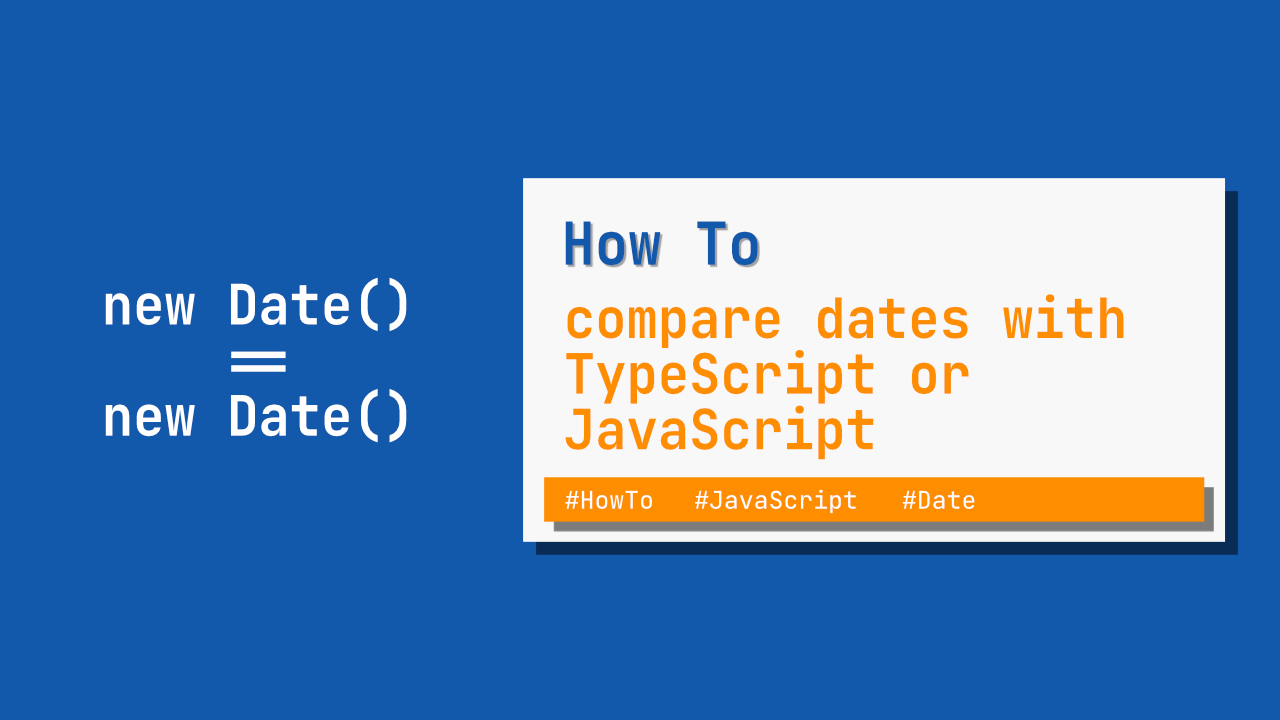
In this tutorial we will learn how to compare dates with TypeScript or JavaScript. Working with dates can be a painful experience but comparing them is not too bad as it works the same way as general object comparisons.
We will start by looking at dates and how they work when we compare them, and then we will look at toISOString()
and getTime()
.
TLDR: To compare dates you can use thetoISOString()
orgetTime()
functions that are build intoDate
. This article just runs through a few examples.
NOTE: You can find the repo for this code here - https://github.com/programmingwithswift/CompareDatesWithTypeScriptOrJavaScript
This is what my file looks like before we start with the comparisons:
const dateOne = new Date('2021-05-10')
const dateTwo = new Date('2021-05-10')
console.log(dateOne)
console.log(dateTwo
Nothing special, we are creating two dates, both the same date, and then we print that to the console so that we can see what the code outputs.
For each section below I have created a function with a few comparison operators so that we can see how these comparisons work.
Direct Date Comparison
As the title for this section says, we will take a look at direct date comparison, i.e comparing the Date
objects directly.
The code that I have looks like this:
function directDateComparison() {
console.log('\ngetTimeDateComparison')
console.log('=====================\n')
// 1. Abstract equality check
if (dateOne == dateTwo) {
console.log('Dates are the same: Abstract equality')
} else {
console.log('Date are not the same: Abstract equality')
}
// 2. Strict equality check
if (dateOne === dateTwo) {
console.log('Dates are the same: Strict equality')
} else {
console.log('Date are not the same: Strict equality')
}
// 3. Greater than check
if (dateOne > dateTwo) {
console.log('dateOne is greater than dateTwo')
} else {
console.log('dateOne is not greater than dateTwo')
}
// 4. Less than check
if (dateOne < dateTwo) {
console.log('dateOne is less than dateTwo')
} else {
console.log('dateOne is not less than dateTwo')
}
}
directDateComparison()
If you run the above function, you should get the follow output(remember, I have those two console logs at the top of the file that will print the two dates):
2021-05-10T00:00:00.000Z
2021-05-09T23:00:00.000Z
getTimeDateComparison
=====================
Date are not the same: Abstract equality
Date are not the same: Strict equality
dateOne is greater than dateTwo
dateOne is not less than dateTwo
Let's run through the code, it will be a super fast run through as there is not much to say after seeing the above output.
1. Abstract equality check
The first check is the abstract equality. This check fails, which makes sense because when comparing two objects together, it will never be true.
2. Strict equality check
This is similar to the abstract equality check, it will also fail because object comparison fails.
3. Greater than check
For some reason I expected the greater than and less that checks to work. The greater than check will be false as well.
4. Less than check
Same as the above, this does not work either.
None of the comparisons that we do on the Date
object will work as expected. So, how do we compare dates in TypeScript or JavaScript? Well, the Date
type has two methods that we can use, namely toISOString()
and getTime()
.
Let's first look at toISOString()
in the next section.
ISO string date comparison
We have taken a look at the direct Date
comparisons, now we will look at using toISOString()
. If you don't want to read the rest of this section, this will work in the same way that normal string comparisons work.
Let's look at the code:
function isoStringDateComparison() {
console.log('\nisoStringDateComparison')
console.log('=====================\n')
// 1. Abstract equality check toISOString()
if (dateOne.toISOString() == dateTwo.toISOString()) {
console.log('dateOne is equal to dateTwo: Abstract equality - toISOString()')
} else {
console.log('dateOne is not equal to dateTwo: Abstract equality - toISOString()')
}
// 2. Strict equality check toISOString()
if (dateOne.toISOString() === dateTwo.toISOString()) {
console.log('dateOne is equal to dateTwo: Strict equality - toISOString()')
} else {
console.log('dateOne is not equal to dateTwo: Strict equality - toISOString()')
}
// 3. Greater than check toISOString()
if (dateOne.toISOString() > dateTwo.toISOString()) {
console.log('dateOne is greater than dateTwo: toISOString()')
} else {
console.log('dateOne is not greater than dateTwo: toISOString()')
}
// 4. Less than check toISOString()
if (dateOne.toISOString() < dateTwo.toISOString()) {
console.log('dateOne is less than dateTwo: toISOString()')
} else {
console.log('dateOne is not less than dateTwo: toISOString()')
}
}
isoStringDateComparison()
If you run the above function, the output should look like this:
2021-05-10T00:00:00.000Z
2021-05-09T23:00:00.000Z
isoStringDateComparison
=====================
dateOne is equal to dateTwo: Abstract equality - toISOString()
dateOne is equal to dateTwo: Strict equality - toISOString()
dateOne is not greater than dateTwo: toISOString()
dateOne is not less than dateTwo: toISOString()
1. Abstract equality check toISOString()
Ah! We see that this will now work, which makes sense because the string value that is returned by toISOString()
will be the same for the dates. As mentioned earlier, this works just like any other string comparison.
2. Strict equality check toISOString()
This is the same as the above, it will work because it is working with a string.
3. Greater than check toISOString()
This works as expected as well. We can use the greater than check to see if one date is greater than another.
4. Less than check toISOString()
This works too, as would be expected if the greater than check works.
We have now seen that if we use toISOString()
we can compare dates easily, no matter if it is an equality check or if it is a greater than/less than check.
Time date comparison
The time comparison will be the last way that we compare dates. We will use the getTime()
function, as before, if you don't want to read this section, this will work in the same way as comparing two numbers.
Let's look at the code:
function getTimeDateComparison() {
console.log('\ngetTimeDateComparison')
console.log('=====================\n')
// 1. Abstract equality check getTime()
if (dateOne.getTime() == dateTwo.getTime()) {
console.log('dateOne is equal to dateTwo: Abstract eqaulity - getTime()')
} else {
console.log('dateOne is not equal to dateTwo: Abstract eqaulity - getTime()')
}
// 2. Strict equality check getTime()
if (dateOne.getTime() === dateTwo.getTime()) {
console.log('dateOne is equal to dateTwo: Strict eqaulity - getTime()')
} else {
console.log('dateOne is not equal to dateTwo: Strict eqaulity - getTime()')
}
// 3. Greater than check getTime()
if (dateOne.getTime() > dateTwo.getTime()) {
console.log('dateOne is greater than dateTwo: getTime()')
} else {
console.log('dateOne is not greater than dateTwo: getTime()')
}
// 4. Less than check getTime()
if (dateOne.getTime() < dateTwo.getTime()) {
console.log('dateOne is less than dateTwo: getTime()')
} else {
console.log('dateOne is not less than dateTwo: getTime()')
}
}
getTimeDateComparison()
If you run the above code, you will get the following output:
2021-05-10T00:00:00.000Z
2021-05-09T23:00:00.000Z
getTimeDateComparison
=====================
dateOne is equal to dateTwo: Abstract eqaulity - getTime()
dateOne is equal to dateTwo: Strict eqaulity - getTime()
dateOne is not greater than dateTwo: getTime()
dateOne is not less than dateTwo: getTime()
1. Abstract equality check getTime()
Just like toISOString
using getTime
works and will compare the dates properly. This makes sense because getTime
will return a number.
2. Strict equality check getTime()
The same goes for a strict equality check as it works with numbers as well.
3. Greater than check getTime()
As expected, a greater than check will work with numbers too, as usual.
4. Less than check getTime()
Last but not least, a less than check will work on a number too.
Conclusion
If we want to compare dates we need to use functions like toISOString
or getTime
to return a data type that we can use with comparison operators, otherwise, trying to compare objects will not work for our purposes.
You can find the full source code here.