How to Convert an object to JSON with Swift
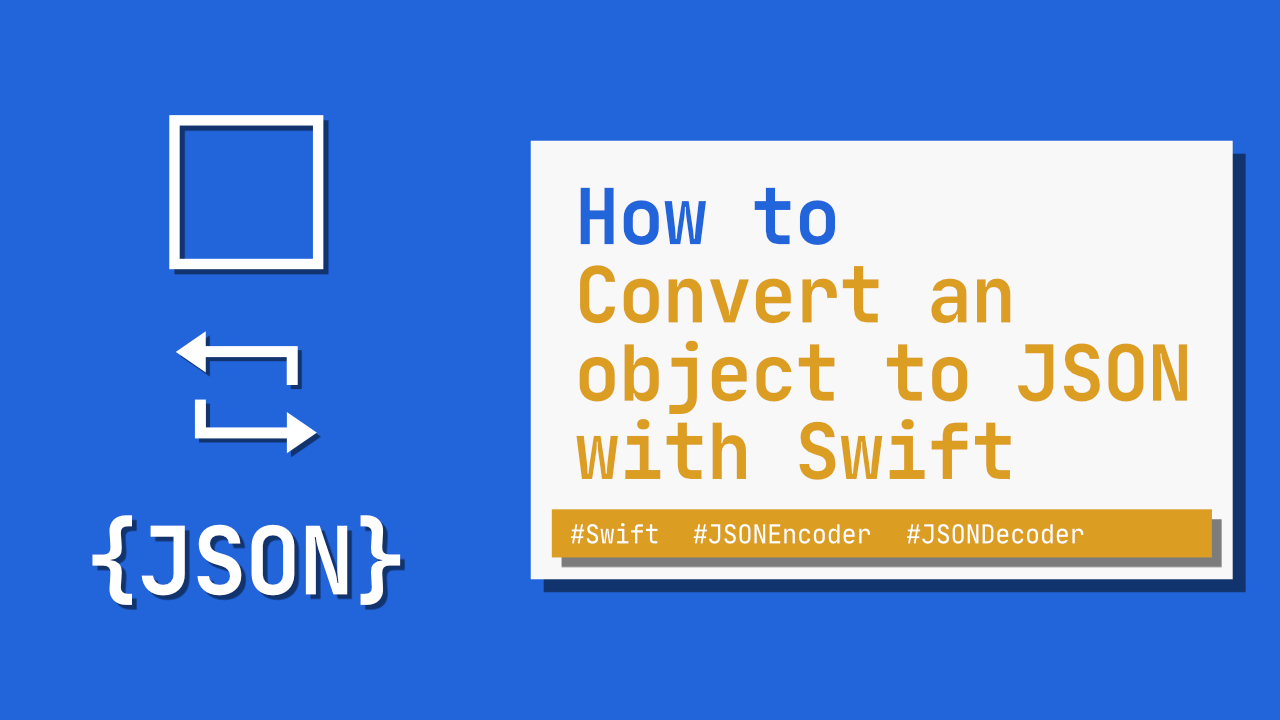
There could be a few reasons why you would want to convert a swift object to JSON. In this tutorial we will take a look at how we can convert a swift object to a JSON string and how to convert the JSON string to a swift object.
First, let's create our struct. This is going to be a simple struct called City
, it will have one property called name
, which will be the name of the city.
Create the following Struct:
struct City: Codable {
let name: String
}
As you can see, this struct adopts the Codable
protocol. If we do not adopt this protocol we will not be able to Encode
and Decode
the struct.
Next, we will create a new instance of City
, add the following code:
let city = City(name: "London")
Convert Object to JSON
Now that we created our struct as well as a new instance of our struct, we can convert this object to JSON.
To do this, we use the following code:
let encodedData = try JSONEncoder().encode(city)
let jsonString = String(data: encodedData,
encoding: .utf8)
On the first line we create a new instance of JSONEncoder
and then we call encode
. encode
requires the argument to of type Encodable
. Our City
struct adopts Codable
which is a type alias for both Encodable
as well as Decodable
.
On the second line we convert the encodedData
to a string. String has an initialiser that allows us to convert Data
to String
. Now that we have converted our object to a string, let's print it out to see what it looks like:
If we add the following code:
print(jsonString)
It will output: Optional("{\"name\":\"London\"}")
, perfect! We have successfully converted our object to a JSON string.
Convert JSON to Object
Now that we have converted our object to JSON, let's convert the JSON back to an object.
To do this we use the following code:
if let dataFromJsonString = jsonString?.data(using: .utf8) {
let cityFromData = try JSONDecoder().decode(City.self,
from: dataFromJsonString)
print(cityFromData.name)
}
As we saw before, String
has an initialiser that will allow us to convert Data
to a string, but it also has a function that will allow us to convert a string to Data
. This method is conveniently called data(using:)
. This data
method will return an optional Data
type, so we will use if let
to unwrap it.
Once the Data
has been unwrapped, we will decode it. Previously when we encoded the object we used JSONEncoder().encode()
, but, when we decode data we need to use JSONDecoder().decode()
. This decode
method will take two arguments. The first is the type that you want to decode your data to, and the second argument is the data itself. As you can see in the code, the type we want to decode to is City
.
JSONDecode().decode()
will return a type of City
, this means that we should be able to access the name
property on city. To test this, we use a simple print
, which should print the following to the console: London
Conclusion
That is how simple it is to convert an object to JSON. Using the built in JSONEncoder
and JSONDecoder
we can easily convert an object to JSON, but we can just as easily convert JSON to an object.
If you want the full source code for this article, you can find it here.