How to convert array to string with Swift
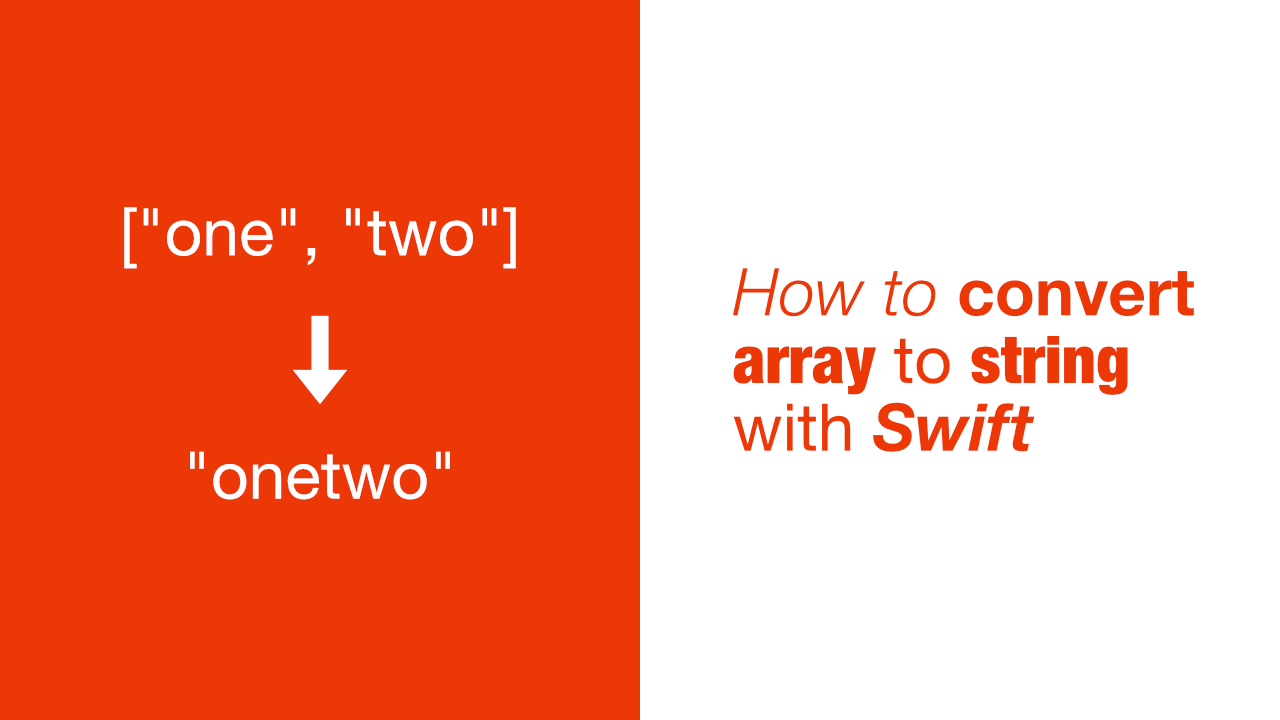
In some situations one might want to convert an array of strings to a string. There are a few ways to do this, all are quick and easy but for most use cases the simplest solution will be the best solution.
Array.joined
Array.joined
is the simplest solution out of the three solutions that I will be going over today. Let's look at the array that we will be using:
var array = ["one", "two", "three", "four", "five"]
All we need to do to convert this to a string is the following:
let joinedArray = array.joined(separator: "")
This will create a string from the array of strings. The separator
is used to specify how you want to separate each string in the array once they are joined. In this case I don't want them to be separated so the output string for the above will be the following: onetwothreefourfive
.
Array.reduce
Shorthand reduce
We can also use Array.reduce
to create a string from a string array. I will be using the same array that I used in Array.joined
.
This is how we can use reduce
:
let reducedArrayShorthand = array.reduce("", +)
As you can see this is quite simple as well, but personally I don't find it as easy to read due to the addition operator that is required for this.
The output for this solution will be the same as Array.joined
.
Long reduce
This is the last way to convert a string array into a string that we will be talking about in this tutorial. I would not suggest doing it this way unless you need to do some processing on each element in the string array before combining them.
Once again, I will be using the same array that I used in Array.joined
, but the code to create a string is the following:
let reducedArray = array.reduce("") { (stringOne, stringTwo) in
// Process strings if required
return stringOne + stringTwo
}
This is basically exactly the same as the short hand example, which is why I would not use this unless I needed to do some extra work with the strings before creating the output string.
This will result in the same output value as in Array.joined
.