How to convert a byte array to String with JavaScript
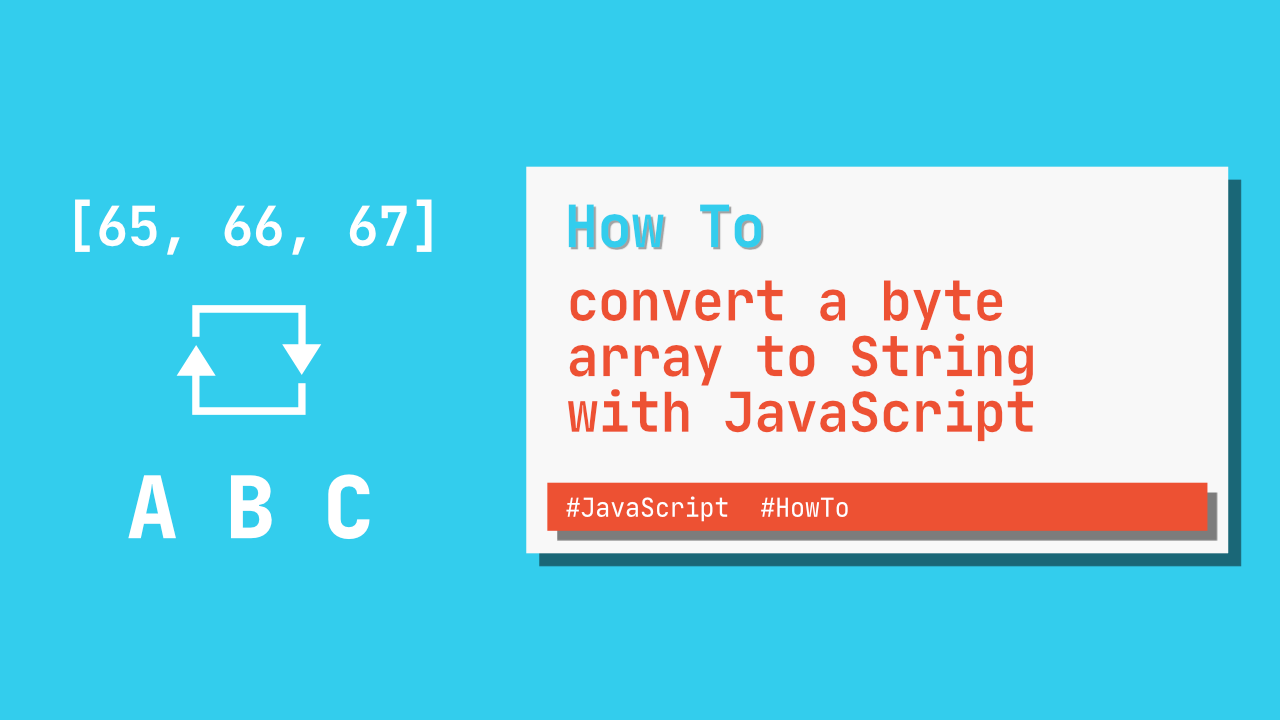
In this tutorial I will show you how you can convert a byte array to a string using JavaScript. I was busy with a task recently that required me to do this, luckily, JavaScript makes this easy with the built in String.fromCharCode()
function.
Let's see how we can use this function:
// 1
const byteArray = [65, 66, 67, 68, 69, 70]
// 2
const bytesString = String.fromCharCode(...byteArray)
// 3
console.log('Bytes to string: ', bytesString)
Let's go over this line by line:
- On line
1
, we simply create a new constant calledbyteArray
and assign array with values from65
to70
. - Line
2
is where the conversion happens. We simply pass inbyteArray
with the spread operator andfromCharCode
will handle the rest. If we didn't want to use the spread operator we could have done,fromCharCode.apply(null, byteArray)
. - The last line is obvious, we just
console.log
, the output will look like this:
Bytes to string: ABCDEF
Converting byte array to JSON
As I mentioned at the beginning of this article, I had a task that required me to use this, but I had to convert a byte array to JSON
.
So, while this is almost identical to the first example, I just want to add it here as an example.
const jsonBytes = [123, 34, 107, 101, 121, 79, 110, 101, 34, 58, 32, 34, 86, 97, 108, 117, 101, 32, 111, 110, 101, 34, 44, 34, 107, 101, 121, 84, 119, 111, 34, 58, 32, 34, 86, 97, 108, 117, 101, 32, 116, 119, 111, 34, 125]
const jsonBytesToString = String.fromCharCode(...jsonBytes)
// 1
console.log("Bytes as a string: ", jsonBytesToString)
// 2
const jsonFromString = JSON.parse(jsonBytesToString)
// 3
console.log('String to JSON: ', jsonFromString['keyOne'])
As you can see, the above is identical apart from the last two lines. In this example we have a bigger byte array, jsonBytes
, but it all works the same.
- We print the output of
fromCharCode
which will look like this:Bytes as a string: {"keyOne": "Value one","keyTwo": "Value two"}
- Next, we parse the string using
JSON.parse
. - Lastly, we output the the value for
keyOne
, which looks like this:String to JSON: Value one
Conclusion
Converting an array of bytes to a string with JavaScript is very easy when we use the fromCharCode
function, however, if you string have special characters you might want to look at a different solution. The reason why this won't work with special characters is due to some special characters using more than one byte, and this solution does not cater for that. While I haven't tested the solution from this link, this article might give you some more insight for this issue.