How to convert Dictionary to Json with Swift
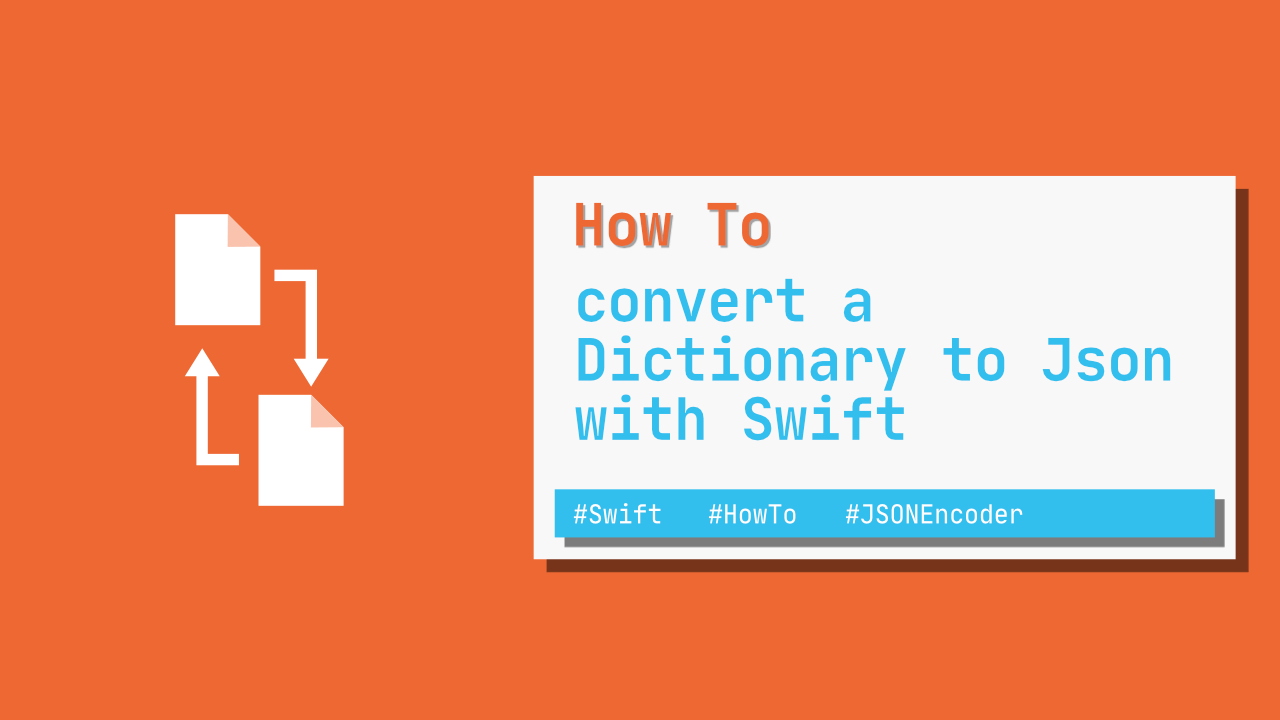
It is a common task to convert a Dictionary
to JSON
. In this tutorial I will show you how you can do just that, luckily for us, Swift makes this incredibly easy.
A quick note before we get started, the dictionary's value
needs conform to Encodable
or Codable
. Codable
is a type alias for Encodable & Decodable
.
The first thing that we are going to do is to create a very simple struct
:
struct MyObject: Codable {
let name: String
}
In the above code we create a struct
called MyObject
. MyObject
will conform to Codable
rather than Encodable
. By adopting Codable
we can encode and decode.
This will depend on how you have designed your application, you might only need one or the other.
Next we add one property, name
, which is a String
. String
is already Codable
so this example will work automatically. If you are using custom types, you might need to make them Codable
.
Next let's create the dictionary:
let dictionary = ["object-1": MyObject(name: "Object 1")]
This is a simple dictionary, we have one key, object-1
, and one value, which is an instance of MyObject
.
Now that we have everything setup, let's convert this to JSON
:
do {
let encodedDictionary = try JSONEncoder().encode(dictionary)
print(encodedDictionary)
} catch {
print("Error: ", error)
}
As you can see, converting from Dictionary
to JSON
is a one liner. All we need to use is JSONEncoder
and then use the built in encode
method. The encode
method takes one parameter. The parameter is generic, but whatever type you are passing in, it needs to adopt Encodable
. In our case we have adopted Codable
so we are covered.
Conclusion
As you can see, converting a Dictionary
to JSON
is super easy, the actual conversion is only one line.
You can find the full source code here.