How to find duplicates in an Array with JavaScript
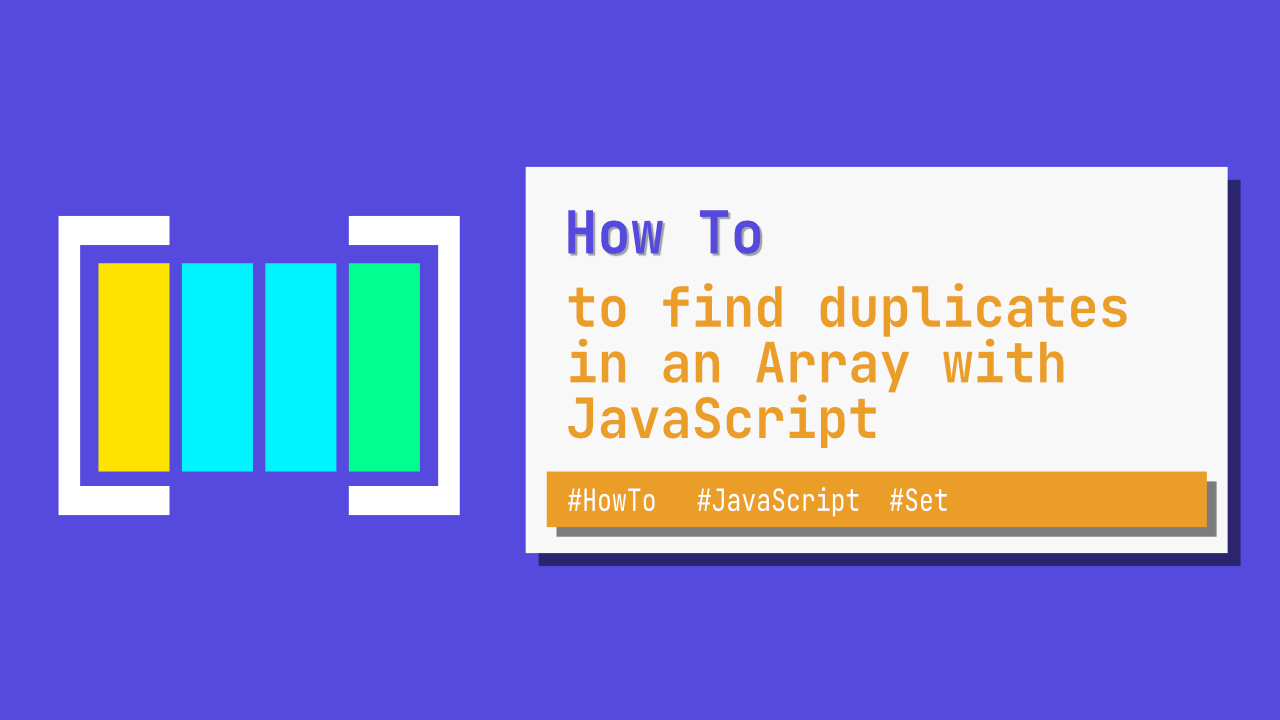
In this tutorial I will show you how you can check if an Array
contains duplicate elements, but also, we will look at how you can return the elements that are duplicates. Both techniques focussed around the Set
data type.
Let's start with the data that will use in both examples:
const shoppingList = [
'bread',
'bread',
'milk',
'pie',
'juice'
]
Option 1: Check if an Array contains duplicate elements
This is the easier of those two methods that I will talk about in this tutorial, and in fact, it is basically a one liner logic wise.
function doesArrayContainDuplicates(list) {
return new Set(list).size < list.length
}
If you run the above code, you should have the following output:
true
This function is pretty straightforward, but I will give a quick rundown.
Basically what this function does is it takes in an array. It will then initialise a Set
from that array and use the size
property which gets compared to the input array's length
. If the Set
has fewer elements, we know that the input array contains duplicate elements.
Option 2: Find and return duplicate Array elements
The following method is more complicated than option 1, however, it is doing more than just returning whether or not an array contains duplicates as it will also return the duplicate values.
Take a look at the following code:
function findAndReturnDuplicatesInArray(list) {
const inputList = new Set()
const duplicates = new Set()
for (const item of list) {
if (inputList.has(item)) {
duplicates.add(item)
} else {
inputList.add(item)
}
}
return duplicates
}
If you run the above function, you should get the following output:
Set(1) { 'bread' }
What is happening here?
We initialise two Set
's, one Set
will be used to keep track of the elements that we have already checked, and the other Set
will be used to keep track of the duplicate elements.
After that, we loop through the list
array that was passed to our function. When looping, it will check if the inputList
Set
contains the current item
, if the Set
does contain the item
then it will add that item
to the duplicates
Set
, if inputList
does not contain the item
, then item
gets added to inputList
At the end of this function we return the duplicates
Set
which will contain all the duplicate values.
Bonus: Remove duplicate elements from Array
Another great use of Set
can be to remove duplicates from an Array
.
To do this, all we need to do is the following:
const shoppingListSet = new Set(shoppingList)
const shoppingListArray = new Array.from(shoppingListSet)
While this is powerful, I am not a fan of using this. I find it to be a bit messy. If I did need this, I would put it in a function just so that it would be cleaner to use.
Conclusion
Finding out whether an Array contains duplicates is super easy when using Set
, likewise, finding and returning the duplicate elements is quite simple too. The Set
data type can be an incredibly powerful tool, one other benefit of using a set is that the has
function could be much faster than the includes
function on the Array data type, depending on the amount of data you have.
If you found this article helpful, please consider sharing it with others that might also find it helpful.
You can find the full source code here.