How to get the index from for-of loop with JavaScript
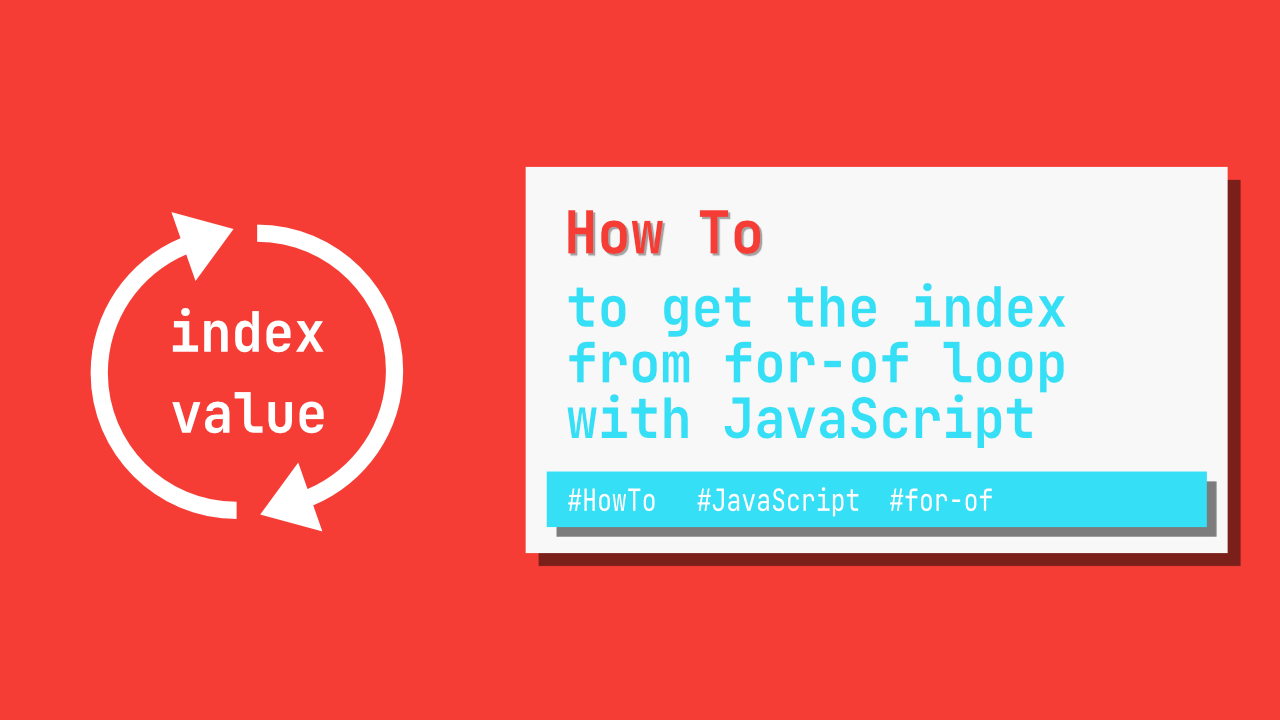
In this tutorial I will show you two ways that you can get the index
when using a for-of
loop.
Let's start with the data that I will be using:
const shoppingList = [
'bread',
'milk',
'pie',
'juice'
]
Option 1: .keys()
The first and easiest way to get the index
with a for-of
loop is to use the Array
's built in keys
function, like this:
for (const index of shoppingList.keys()) {
console.log(`${shoppingList[index]} at ${index}`)
}
If you run the above code, you should have the following output:
bread at 0
milk at 1
pie at 2
juice at 3
Option 2: .entries()
This is my preferred way to get the index
in a for-of
loop. The main reason why I prefer this way is because it provides additional data, rather than changing the way that I use for-of
normally.
In the below code you can see what I mean:
for (const [index, shoppingItem] of shoppingList.entries()) {
console.log(`${shoppingItem} at ${index}`)
}
Normally with a for-of
loop you get the element value and not the element index. But using entries
you can get both.
If you run the above code, you should have the following output:
bread at 0
milk at 1
pie at 2
juice at 3
Conclusion
I found getting the index
in a for-of
loop a bit unintuitive, but, after playing around with it a bit, it is quite straight forward.
If you found this article helpful, please consider sharing it with others that could also find it helpful.
You can find my full code here.