How to ignore/skip or run only one test with Jest
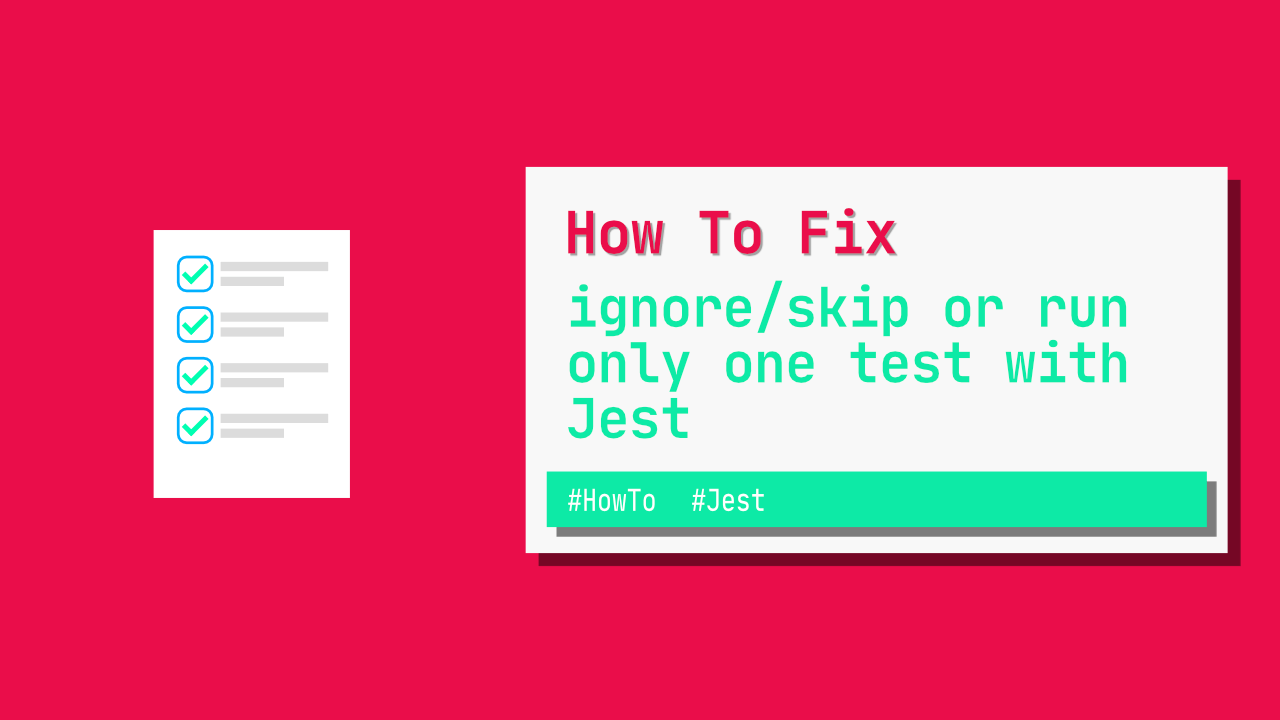
In this tutorial I will show you how you can skip certain tests, or, run only specific tests with Jest. Recently I needed to do this at work, but I always used to comment things out, which can become frustrating, but then I found out that one can skip or only run specifics tests.
You can find the full source code for the below examples here.
How to skip a test or multiple tests
In the below group of tests, I have three tests. test 1
and test 3
will run, but because test 2
uses test.skip
and not just test
, it will not run. Once the tests have completed, Jest will will output what tests have run and which have been skipped.
describe('A: Skip test 2', () => {
test('A: test 1', () => {
console.log('A: test 1 complete')
})
test.skip('A: test 2', () => {
console.log('A: test 2 complete')
})
test('A: test 3', () => {
console.log('A: test 3 complete')
})
})
If we run the above test, the output will look something like:
PASS __tests__/index.js
A: Skip test 2
✓ A: test 1 (7 ms)
✓ A: test 3 (1 ms)
○ skipped A: test 2
console.log
A: test 1 complete
at Object.<anonymous> (__tests__/index.js:3:13)
console.log
A: test 3 complete
at Object.<anonymous> (__tests__/index.js:11:13)
Test Suites: 1 passed, 1 total
Tests: 1 skipped, 2 passed, 3 total
Snapshots: 0 total
Time: 0.888 s, estimated 1 s
Ran all test suites.
How to skip all tests in describe
Using skip
with describe
works in the same way as test
does in this case. All we need to do in order to skip the tests in describe
is to use describe.skip
:
describe.skip('B: Skip group B entirely', () => {
test('B: test 1', () => {
console.log('B: test 1 complete')
})
test('B: test 2', () => {
console.log('B: test 2 complete')
})
test('B: test 3', () => {
console.log('B: test 3 complete')
})
})
After we run the above tests, we will get the following output:
Test Suites: 1 skipped, 0 of 1 total
Tests: 3 skipped, 3 total
Snapshots: 0 total
Time: 0.974 s, estimated 1 s
Ran all test suites.
How to run only certain describe groups or tests
only
is basically the opposite of skip
, but it should only be used when debugging. The reason being is that it makes it easier to debug large test files. Anyway, let's take a look at the code. Just before we do, I have included test A
and B
so that we can see what the output looks like now when we use only
.
describe('A: Skip test 2', () => {
test('A: test 1', () => {
console.log('A: test 1 complete')
})
test.skip('A: test 2', () => {
console.log('A: test 2 complete')
})
test('A: test 3', () => {
console.log('A: test 3 complete')
})
})
describe.skip('B: Skip group B entirely', () => {
test('B: test 1', () => {
console.log('B: test 1 complete')
})
test('B: test 2', () => {
console.log('B: test 2 complete')
})
test('B: test 3', () => {
console.log('B: test 3 complete')
})
})
describe.only('C: Only run this suite', () => {
test('C: test 1', () => {
console.log('C: test 1 complete')
})
test('C: test 2', () => {
console.log('C: test 2 complete')
})
test('C: test 3', () => {
console.log('C: test 3 complete')
})
})
After running the above tests, this output is the following:
PASS __tests__/index.js
A: Skip test 2
○ skipped A: test 1
○ skipped A: test 2
○ skipped A: test 3
B: Skip group B entirely
○ skipped B: test 1
○ skipped B: test 2
○ skipped B: test 3
C: Only run this suite
✓ C: test 1 (9 ms)
✓ C: test 2 (1 ms)
✓ C: test 3 (1 ms)
console.log
C: test 1 complete
at Object.<anonymous> (__tests__/index.js:34:13)
console.log
C: test 2 complete
at Object.<anonymous> (__tests__/index.js:38:13)
console.log
C: test 3 complete
at Object.<anonymous> (__tests__/index.js:42:13)
Test Suites: 1 passed, 1 total
Tests: 6 skipped, 3 passed, 9 total
Snapshots: 0 total
Time: 1.015 s
Ran all test suites.
As we can see from the above, when we use only
, it will skip everything else that is outside of only
. But, let's take a look at another example, test D
, with test A
, B
and C
included:
describe('A: Skip test 2', () => {
test('A: test 1', () => {
console.log('A: test 1 complete')
})
test.skip('A: test 2', () => {
console.log('A: test 2 complete')
})
test('A: test 3', () => {
console.log('A: test 3 complete')
})
})
describe.skip('B: Skip group B entirely', () => {
test('B: test 1', () => {
console.log('B: test 1 complete')
})
test('B: test 2', () => {
console.log('B: test 2 complete')
})
test('B: test 3', () => {
console.log('B: test 3 complete')
})
})
describe.only('C: Only run this suite', () => {
test('C: test 1', () => {
console.log('C: test 1 complete')
})
test('C: test 2', () => {
console.log('C: test 2 complete')
})
test('C: test 3', () => {
console.log('C: test 3 complete')
})
})
describe('D: Only run test 2', () => {
test('D: test 1', () => {
console.log('D: test 1 complete')
})
test.only('D: test 2', () => {
console.log('D: test 2 complete')
})
test('D: test 3', () => {
console.log('D: test 3 complete')
})
})
If we run the above, we get the following output:
PASS __tests__/index.js
A: Skip test 2
○ skipped A: test 1
○ skipped A: test 2
○ skipped A: test 3
B: Skip group B entirely
○ skipped B: test 1
○ skipped B: test 2
○ skipped B: test 3
C: Only run this suite
✓ C: test 1 (7 ms)
✓ C: test 2 (1 ms)
✓ C: test 3 (1 ms)
D: Only run test 2
✓ D: test 2 (1 ms)
○ skipped D: test 1
○ skipped D: test 3
console.log
C: test 1 complete
at Object.<anonymous> (__tests__/index.js:34:13)
console.log
C: test 2 complete
at Object.<anonymous> (__tests__/index.js:38:13)
console.log
C: test 3 complete
at Object.<anonymous> (__tests__/index.js:42:13)
console.log
D: test 2 complete
at Object.<anonymous> (__tests__/index.js:52:13)
Test Suites: 1 passed, 1 total
Tests: 8 skipped, 4 passed, 12 total
Snapshots: 0 total
Time: 0.996 s, estimated 1 s
Ran all test suites.
That is interesting. We can run multiple only
tests. If we use describe.only
, all the tests inside it will run, provided they are not test.skip
. We can also use test.only
, which allows us to run specific tests for debugging.
Conclusion
Being able to run or skip specific test is great, I much prefer using the above techniques rather than commenting tests out.
You can find the full source code for the below examples here.