How to pretty-print JSON with JavaScript
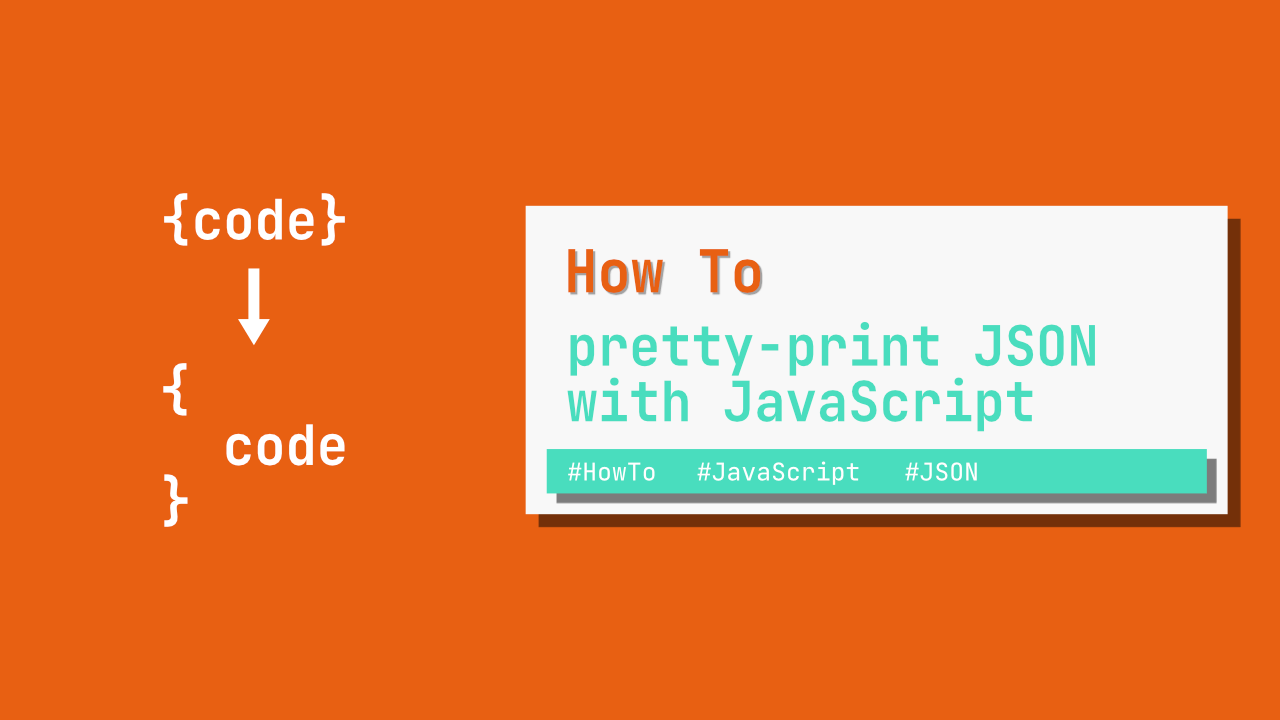
In this tutorial I will show you how you can pretty print a JSON string. It is a one liner, but it makes all the difference.
Normally when we use JSON.stringify
, we only want to serialise a JSON object, but sometimes after we serialise it, we might want to do debugging, or maybe we want to store the JSON with indentation.
JSON.stringify
can take 3 arguments. The first is the JSON object that we want to serialise. The second is a function that changes the serialization process. The third can be a number or a string depending on what you are wanting to achieve.
If you want more infomation on JSON.stringify
you can take a look at the Mozilla developer docs.
Let's first take a look at what normally happens when you try to print a JSON string in the browser or with node.
Before that, let's first look at the JSON string:
const test = JSON.stringify({
"carBrands": [
"Toyota",
"Nissan",
"Volkswagen",
"Fiat"
]
})
If I do a simple console.log
with that in Node or the browser, I get the following output:
{"carBrands":["Toyota","Nissan","Volkswagen","Fiat"]}
If we make use of the other arguments of JSON.stringify
, as we do below, we can change the output:
const test = JSON.stringify({
"carBrands": [
"Toyota",
"Nissan",
"Volkswagen",
"Fiat"
]
}, null, 2)
If we run the above code with node or in the browser using console.log
, we will now get the following:
{
"carBrands": [
"Toyota",
"Nissan",
"Volkswagen",
"Fiat"
]
}
Conclusion
Adding indentation to a JSON is surprisingly simple, but what I find more interesting is the additional functionality that JSON.stringify
could provide if one needed it.