How to replace characters in string with Swift
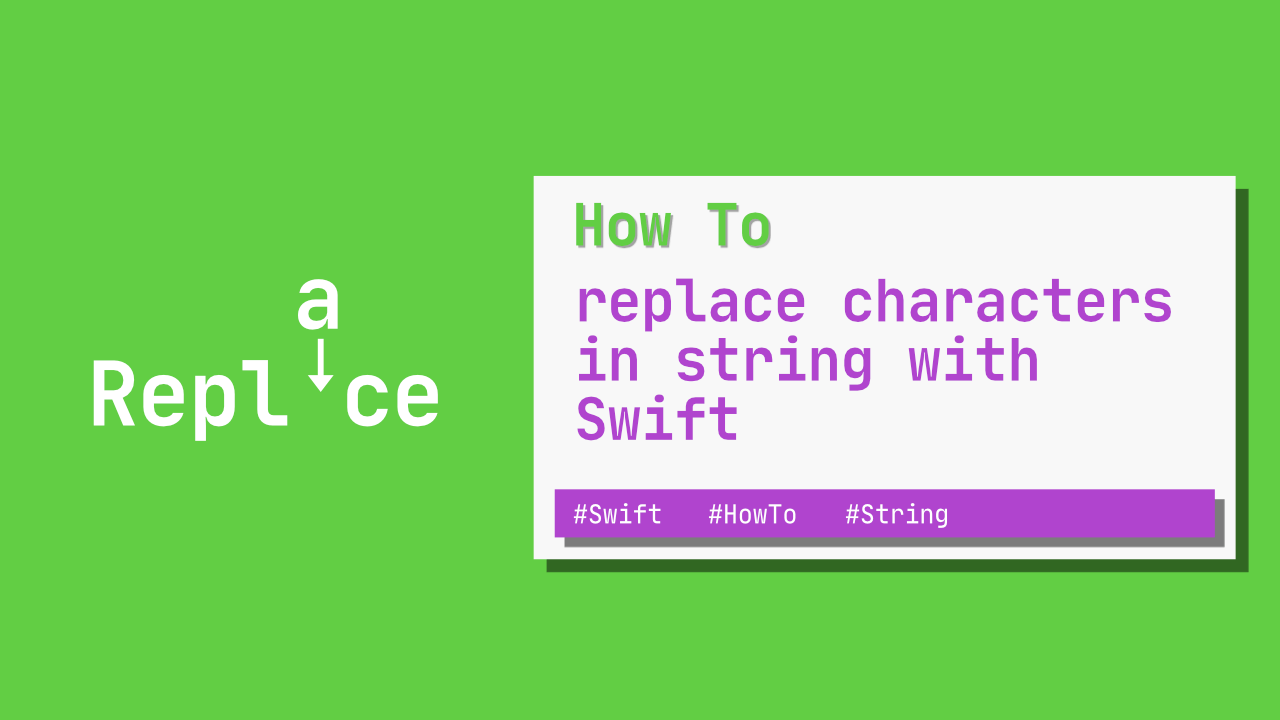
In this tutorial you will learn how to replace characters in a String using Swift. We will be using the following methods replacingOccurrences
, replacingCharacters
as well as replaceSubrange
.
Replace characters with replacingOccurrences method
The first method we are going to look at is replacingOccurrences
. Two things to note about this method.
replaceOccurrences
is not mutating, so it will return a newString
and leave the original as is.- This there are two signatures for this function, we will look at both. The first signature that we will look at will replace all occurrences of the characters you want to replace, the second signature will allow you to pick what you want to replace.
NOTE: The first signature that we will look calls the second signature.
Let's take a look at the code:
let testString = "This is a test string"
var testStringReplacedOccurences = testString.replacingOccurrences(of: "is",
with: "*")
print("testStringReplacedOccurences:", testStringReplacedOccurences)
// OUTPUT: Th* * a test string
As you can see in the above code example, the replaceOccurrences
replaces both occurrences of is
from the testString
. This method is extremely simple to use as all that it requires is the string you want to replace, with the string you want to replace it with.
Now, let's take a look at the second signature for replaceOccurrences
:
let testString = "This is a test string"
var testStringOccurences2 = testString.replacingOccurrences(of: #"\bis"#,
with: "was",
options: .regularExpression,
range: nil)
print("testStringOccurences2:", testStringOccurences2)
// OUTPUT: This was a test string
This is a bit of a different example because I am using a regular expression, but I have done this to show you how powerful this can be.
In the above code example, the output is quite different. Instead of replacing all occurrences of is
, it has only replaced the occurrence where is
was a stand alone word. This is due to this signature allowing us to provide options
, and in the above example, I have used the regularExpression
option.
NOTE: You can find more information on regular expressions in Swift from the Apple Docs
I haven't used the range
parameter in this example, but, if you wanted to, you could use the range with no options. Options is not optional, but you can pass an empty array as its value.
Replace characters with replacingCharacters method
replacingCharacters
is a bit different to replacingOccurrences
, in that it wants us to pass in a range, which will be the characters to be replaced, and then the string that will be used to as a replacement.
This method with also return a new string and not mutate the string that you are using.
Let's take a look at the code:
let testString = "This is a test string"
if let range = testString.range(of: "is") {
let testString2 = testString.replacingCharacters(in: range,
with: "at")
print("testString2: ", testString2)
// OUTPUT: That is a test string
}
The first thing that we need to do is get the range that we are going to use. To get the range, we can simply use String
's built in range
method.
Next we can use replaceCharacters
method. All we need to do is pass in the range that we have, and the string that want to use as the replacement string.
Also, an obvious point, but, when using a range you will be targeting specific characters rather than occurrences of characters.
Replace characters with replaceSubrange method
This is the only method in this tutorial that will mutate the original string, so if that is what you are looking for then this is your only option out of the methods that we have taken a look at.
Let's take a look at the code:
var substringReplace = "Replace a substring"
let endIndex = substringReplace.index(substringReplace.startIndex,
offsetBy: 8)
substringReplace.replaceSubrange(...endIndex,
with: "Replaced the")
print("substringReplace", substringReplace)
// OUTPUT: Replaced the substring
The first difference with this example is that we are using a variable rather than a let. This is due to this method being a mutating method.
Next, we get the end index. The end index is using the start index and we offset it by 8.
Finally, we pass through the range, ...endIndex
, and then we pass through the replacement string. The range will be 8 characters in this example.
Conclusion
Swift has a few ways to replace characters in a string, but my preferred way is replacingOccurrences
. It can be super simple and by default it replaces multiple occurrences, but it can also be very customisable and powerful.
You can find the full source code here.