How to save a file locally with Flutter(Image, Text)
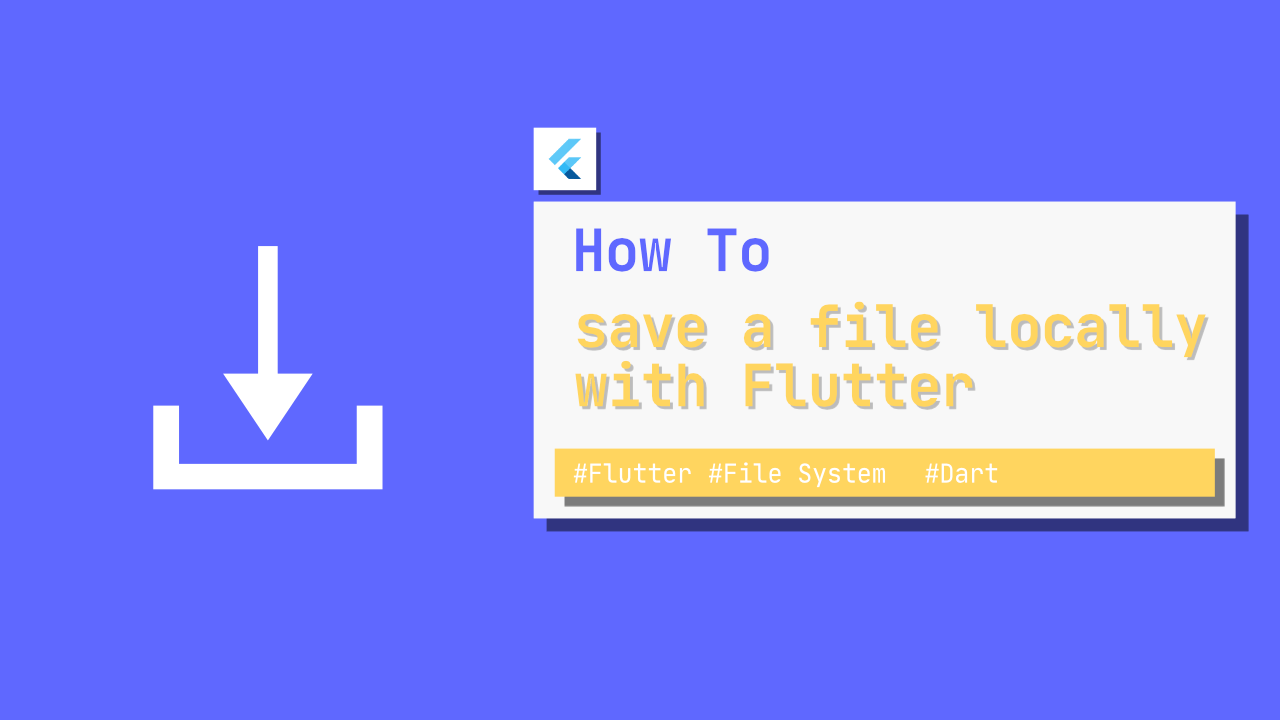
In this tutorial you will learn how you can save a file locally. Flutter has a built in type called File
which will allow us to read and write to a file locally. To make our lives easier we do need to add a package, the path_provider
package, which you can find here.
The path_provider
package is used for "finding commonly used locations on the filesystem".
Before we get started, be sure to add the following to your pubspec.yaml under dependencies:
path_provider: ^1.6.14
Once you have added that to your pubspec, you can run flutter pub get
, after that has completed remember to import the following where ever it is required:
import 'package:path_provider/path_provider.dart';
import 'dart:io';
Step 1: Get the commonly used file path
The first thing that we need to do is to write a function that will return the path to the file that we want to save to. In this example I will be using a hardcoded file name, demoTextFile.txt
but you can update this to whatever you want.
Below the is the code to retrieve the file path:
Future<String> getFilePath() async {
Directory appDocumentsDirectory = await getApplicationDocumentsDirectory(); // 1
String appDocumentsPath = appDocumentsDirectory.path; // 2
String filePath = '$appDocumentsPath/demoTextFile.txt'; // 3
return filePath;
}
- We call
getApplicationDocumentsDirectory
. This comes from thepath_provider
package that we installed earlier. This will get whatever the common documents directory is for the platform that we are using. - Get the path to the documents directory as a
String
- Create the full file path as a
String
.
Step 2: Write content and save the file
Now that we have the getFilePath
function written, we can use that to create a new instance of File
and then use that to write some text to the file.
To do this we need to add the following saveFile
function:
void saveFile() async {
File file = File(await getFilePath()); // 1
file.writeAsString("This is my demo text that will be saved to : demoTextFile.txt"); // 2
}
- Initialise
File
with the file path that gets returned by thegetFilePath
function. - We write and save to the file.
File
has a built in function calledwriteAsString
that will allow us to write aString
to the file.
Step 3: Read the file
Now that we have written to the file, let's see if it worked as expected. Add the following readFile
function to your code:
void readFile() async {
File file = File(await getFilePath()); // 1
String fileContent = await file.readAsString(); // 2
print('File Content: $fileContent');
}
- Once again we initialise
File
with the file path that we used before. - Previously we used the
writeAsString
function that is built in toFile
, but now we can use thereadAsString
function that is also built in toFile
.
Lastly we print the contents of the file that we read from.
Conclusion
Saving to file locally is very easy with Flutter, as long as you can create an instance of file you can write the data.
The full source code for this project can be found here.