How to save data to file with Swift
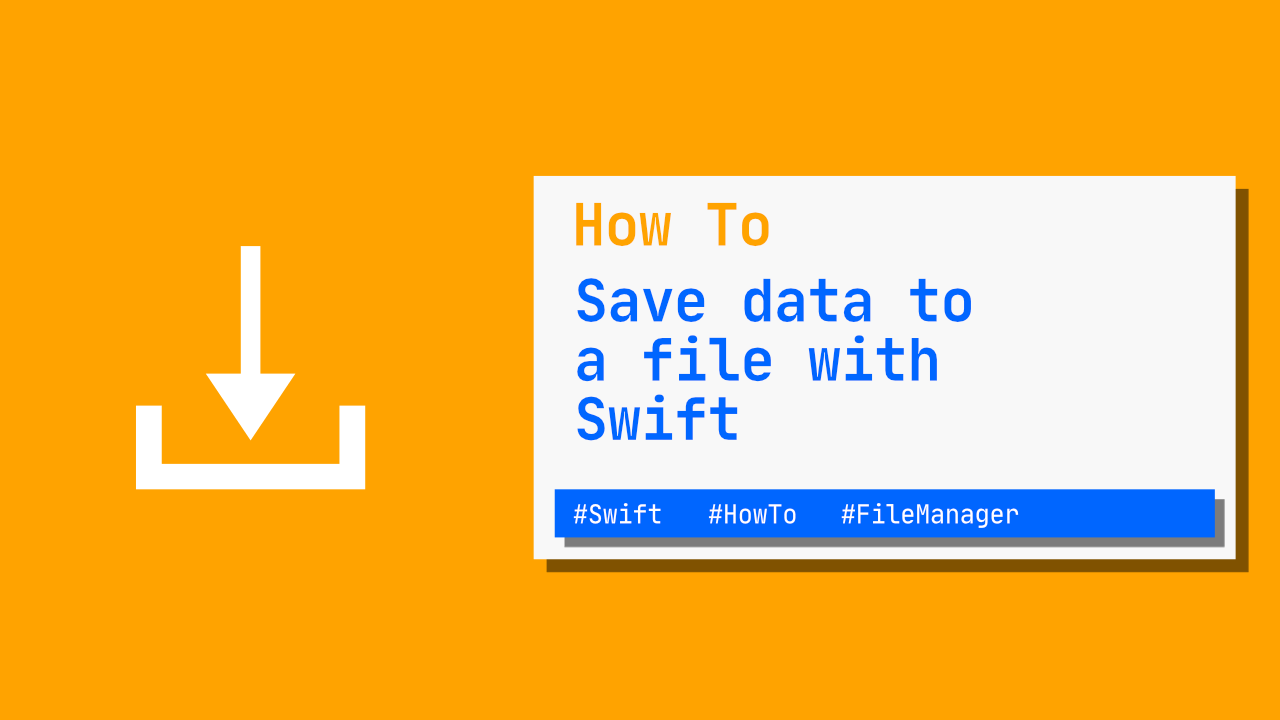
There are many reasons that we might need to save data to a file as an app developer. We might need to save an image, or text or something else.
In this tutorial I will show you how we can save a string to a file, but this can be used on any object whose type is Data
.
Write data to file using write(to:)
This is the code to save data to a file:
let stringToSave = "The string I want to save"
let path = FileManager.default.urls(for: .documentDirectory,
in: .userDomainMask)[0].appendingPathComponent("myFile")
if let stringData = stringToSave.data(using: .utf8) {
try? stringData.write(to: path)
}
So, what is happening in the above code?
The first thing that we are doing is creating a string called stringToSave
. As the name suggests, this is the string that we are going to save to a file.
Next we get the path to the file that we want to write to. To do this we have created a path
constant which uses the FileManager
to get the url for the documentDirectory
. We get the first url in the array and append a path component, in this case the path component is myFile
.
Lastly, we convert convert stringToSave
to data, stringData
. The String
type has a built in method called data
that will allow us to convert the string to data. This data
method takes one argument to specify the string encoding.
Once we have the data we can use the write
method that is found on the Data
type. The write
method takes one argument, the path to the file that we want to write the data to. We already have the path so we will use that.
Conclusion
And that is it! That is all that is needed to write data to a file using Swift. I have a playground file containing the above code as well as code that will read the data from the file. You can find the playground here.