How to save an image to file with Swift
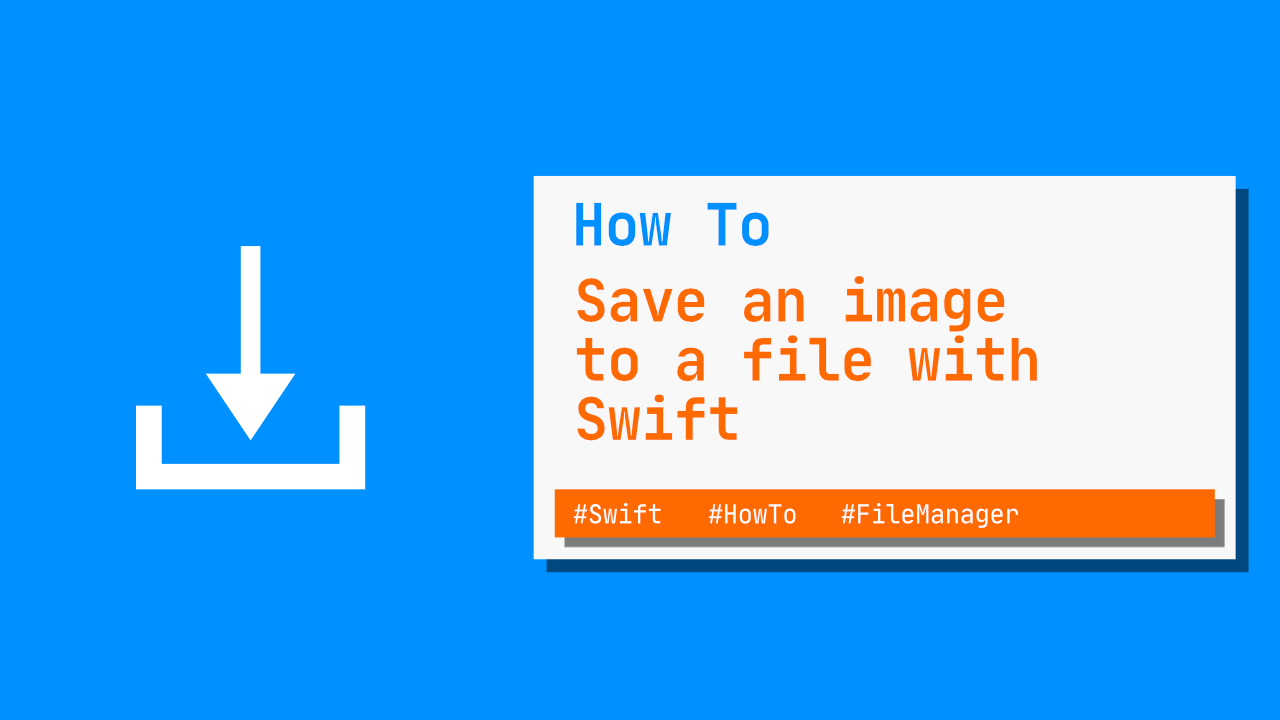
There are numerous reasons to save an image to a file using Swift. In this tutorial I will show how you can do that with just a few lines of code.
Before we get started I want to add a method that we will use in both saving of a png and a jpeg file, this is more of a helper method for this tutorial:
func documentDirectoryPath() -> URL? {
let path = FileManager.default.urls(for: .documentDirectory,
in: .userDomainMask)
return path.first
}
The above code will return an optional URL
to the documents directory.
Save image as PNG
To save an image as a png we can use the pngData
method on UIImage
. pngData
will return Data?
. Data
has a write
method that we will use in order to write the data to a file. Let's see how this will be done:
func savePng(_ image: UIImage) {
if let pngData = image.pngData(),
let path = documentDirectoryPath()?.appendingPathComponent("examplePng.png") {
try? pngData.write(to: path)
}
}
In the above code we have a few things going on. First, we have a method called savePng
. This method will take a UIImage
as an argument.
Now that we have the image, can get call the pngData
method on it. This will return Data?
, in this tutorial we will unwrap it, but you don't have to. After we have the image data we need to get the path to the file we want to save.
This is where we use the documentDirectoryPath
method that I mentioned in the beginning of this tutorial. The documentDirectoryPath
will return an optional URL
. Now that we have the optional url, we can append our file name to it, in this case the file name will be examplePng.png
.
Lastly, if all of the above can be unwrapped, we will call the write
method on pngData
. This write
method will take the path we created earlier as its argument.
The write method can throw an error which is why we are using try?
.
Save image as JPEG
Saving a jpeg image is just as easy as saving a png. UIImage
has a built in method called jpegData
which will allow us to convert our UIImage
to jpeg data.
To save a jpeg file we will use the following code:
func saveJpg(_ image: UIImage) {
if let jpgData = image.jpegData(compressionQuality: 0.5),
let path = documentDirectoryPath()?.appendingPathComponent("exampleJpg.jpg") {
try? jpgData.write(to: path)
}
}
This code very similar to savePng
above. We have a method called saveJpg
which will take a UIImage
as an argument. On the UIImage we call jpegData
, jpegData
requires us to pass through a compressionQuality
argument. In the above example I set it to 0.5
but you can set it to anything you want within 0-1
.
Next we get the path to the directory document and at the same time we append the image file name. For this example I have named the image exampleJpg.jpg
.
Lastly, we make use of the write
method that can be found on jpgData
. This write
method takes in a path as an argument. We will pass in the path that we unwrapped.
As mentioned before, write
can throw, so we need to use try?
when calling it.
Conclusion
Thanks to Data
for having a write method built in and UIImage
being able to return png or jpeg data, Swift makes it incredibly easy to save an image as a file.
If you want to see the full source code, you can find it here. The full source code also has a read method to ensure that the files have been saved.