How to save/write Json to file with Swift
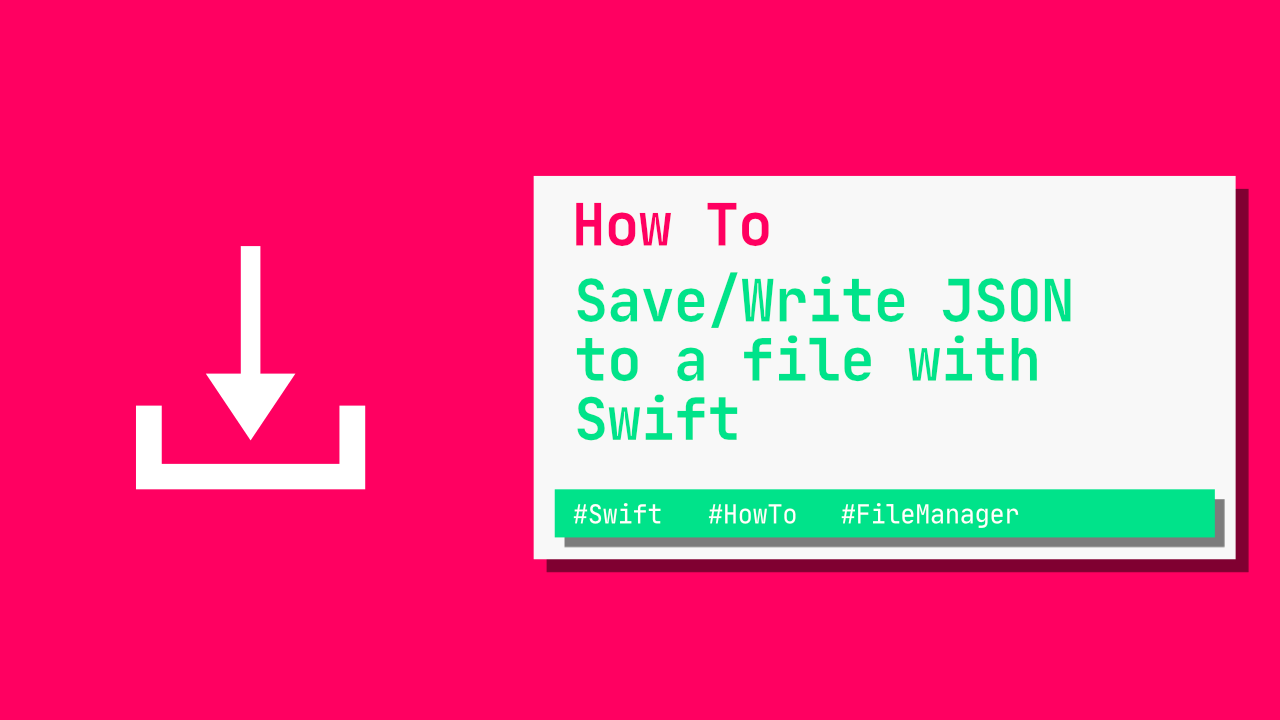
In this tutorial I will show you two methods that you can use to write JSON data/string to a file using Swift.
Save JSON string to file
Saving a JSON string will be the same as saving any other kind of string. Since Swift will see it as a String
object, we can use the write(to:)
method that is built into String
.
let jsonString = "{\"location\": \"the moon\"}"
if let documentDirectory = FileManager.default.urls(for: .documentDirectory,
in: .userDomainMask).first {
let pathWithFilename = documentDirectory.appendingPathComponent("myJsonString.json")
do {
try jsonString.write(to: pathWithFilename,
atomically: true,
encoding: .utf8)
} catch {
// Handle error
}
}
What are we doing above?
The first thing we are doing is creating a mock jsonString
, we will use this in the above code as well as in the Save JSON Data
section below.
Once we have the JSON string we need to get the path to our documents directory. We do this by using FileManager.default.urls
as you can see above. The first URL
in the array that gets returned is what we want.
Now that we have the document directory url, we need to get the file path, pathWithFileName
. To do this we append a filename onto document directory path. In this case I am going to call the file, myJsonString.json
.
Lastly we need to write to the file. String
has a built in method called write(to:)
. This will allow us to write the value of jsonString
to pathWithFilename
. The first argument that write(to:)
expects is the pathWithFilename
, next it requires us to specify if it should be atomic(this has to do with concurrency) and lastly we pass through the encoding that we want to use.
If nothing fails we will have written the jsonString
contents to a file!
Save JSON data to file
Saving JSON data is exactly the same as saving normal data. Data
has a built in method called write
. In fact, saving data and saving a string is very similar.
This is how we can save data(Note: jsonString
is being used from the above section):
if let jsonData = jsonString.data(using: .utf8),
let documentDirectory = FileManager.default.urls(for: .documentDirectory,
in: .userDomainMask).first {
let pathWithFileName = documentDirectory.appendingPathComponent("myJsonData")
do {
try jsonData.write(to: pathWithFileName)
} catch {
// handle error
}
}
If you have read the previous section on how to save a JSON string to a file then this section is very similar, if you only came to see how to save JSON data, then I will go through the above code now.
In the first line we are converting a JSON string, jsonString
, to data. String has a built in method that allows us to convert it to Data
.
Next we get the document directory. To do this we use FileManager.default.urls
. This will return an array, we only want the first item in the array.
Now that we have the document directory we will create a constant called, pathWithFileName
. We will use this to append a file name to the documentDirectory
. I have called this file myJsonData
.
Lastly we can save the file. Data
has a built in method called write(to:)
. This method takes a URL
as an argument. We will pass in pathWithFileName
as our url since that represents the full path to the file that we want to write to.
You should now be able to write JSON data to a file.
Conclusion
Whether you are trying to write a String
or Data
object to a file, Swift makes this easy. Both of them have write(to:)
methods, while the String
version takes a few more arguments, they are very similar in general use. If you wanted to, you could convert the String
to Data
.
You can find the full source code here.