How to sort a dictionary by value with Swift
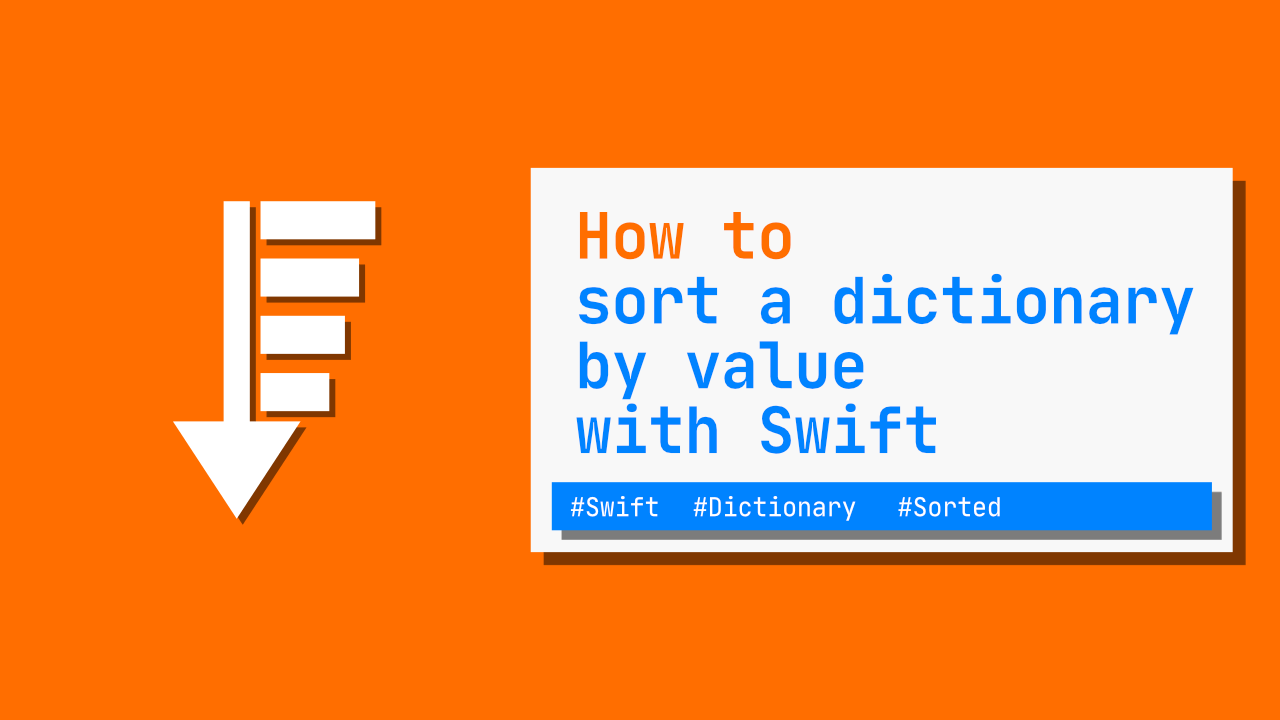
Every now and then you might need to sort a dictionary by value, luckily, dictionary comes with a sorted
method built in.
I am going to use the following dictionary as an example:
let dictionary = [
"a": "A",
"b": "B",
"c": "C",
"d": "D",
"e": "E",
]
Let's sort the dictionary in descending order based on the values:
let sortedOne = dictionary.sorted { (first, second) -> Bool in
return first.value > second.value
}
As you can see, the sorted
method takes a closure as an argument. This closure takes two arguments and returns a bool value. Each argument is a tuple that contains two values, key
and value
. This is what allows us to access the value of the either the key
or value
in the dictionary. Because we want to sort by value
, we will use the value
element.
If we print sortedOne
we will see the following:
[(key: "e", value: "E"), (key: "d", value: "D"), (key: "c", value: "C"), (key: "b", value: "B"), (key: "a", value: "A")]
If you want to sort by ascending order you can use <
instead of >
.
There is a shorter way of sorting by dictionary value. It is basically the same as the previous example, but it is a shorthand version as you can see below:
let sortedTwo = dictionary.sorted {
return $0.value > $1.value
}
Instead of naming the arguments in the closure, we can access them by using $0
and $1
. In this example, $0
is the same as first
and $1
is the same as second
from the first example, sortedOne
.
The tuple arguments have named elements, key
and value
, but, if you wanted to, you could access the elements by position. So instead of $0.value
you could do $0.1
, see the below modified version of sortedTwo
:
let sortedTwo = dictionary.sorted {
return $0.1 > $1.1
}
Personally I am not a fan of this as I find it difficult to read, but if you want the code to be a bit shorter or want to put it on one line, then this can help.
Conclusion
Sorting a dictionary by value is incredibly easy with the sorted
method. And as a quick reminder, <
will sort in ascending order and >
will sort in descending order.
If you want the full source, you can find it here.