How to store a date in UserDefaults with Swift
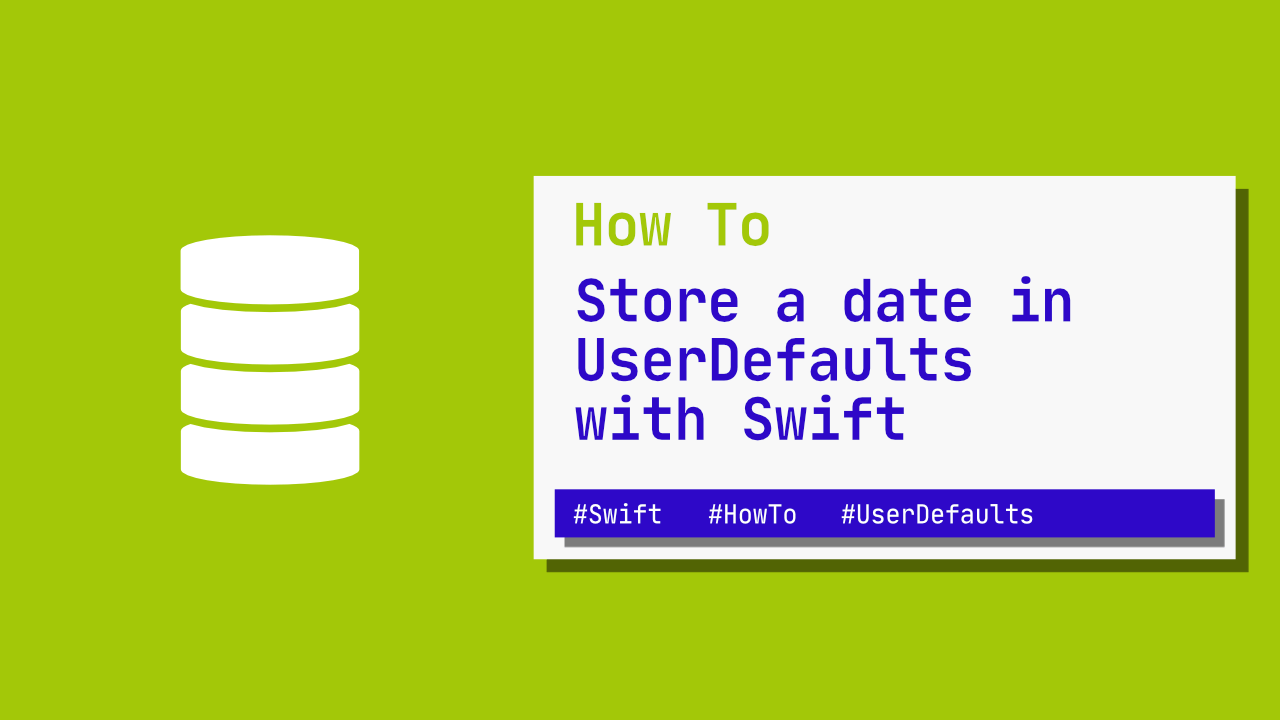
UserDefaults can be a useful tool to store small amounts of information. In this tutorial I will show you how you can store a Date in UserDefaults using Swift.
Storing a date in UserDefaults
Below is the code that we will use to save the date:
let defaults = UserDefaults.standard
defaults.set(Date(), forKey: "currentDate")
if let storedDate = defaults.object(forKey: "currentDate") as? Date {
print("Stored Date: ", storedDate)
}
Storing a date is the same as storing another type. UserDefaults has a few methods for setting a value to a key, one of them allows the value to be of type Any?
. This is what will be used in the above example by default.
In the first line I have created a constant so that I don't have to type UserDefaults.standard
all the time.
Next we will add a value to UserDefaults. To do this we call the .set(value:, forKey:)
method. The value
in this case will be a new instance of Date
and the key will be currentDate
.
Lastly we can retrieve the value from UserDefaults. Because UserDefaults does not support Date
in the same way it does other types, we need to cast the returned value to a Date
. Once we have the returned value we print it out.
If you want the full source code, you can find it here.