How to Use UserDefaults Suites With Swift
Learn what suites are in UserDefaults as well as how to use them.
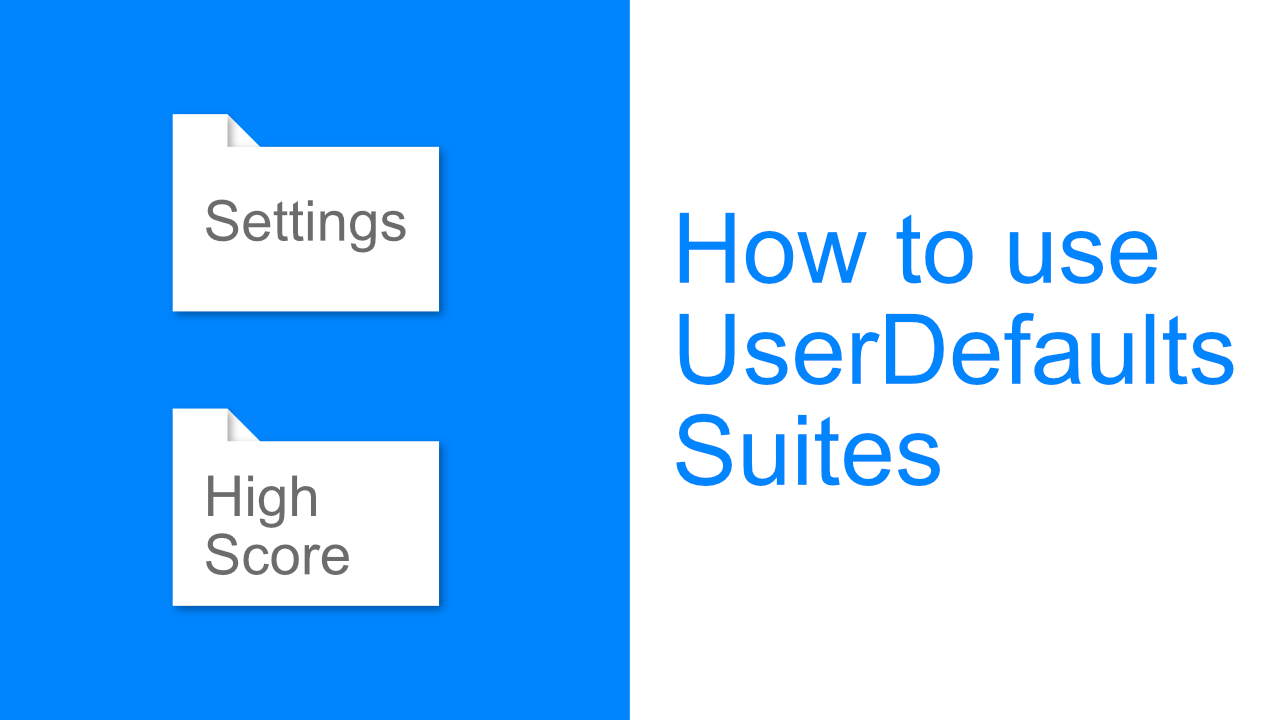
What are UserDefaults suites
UserDefaults
contains different suites that are identified by their suiteName
. This can allow you to create different UserDefaults
for different sections of your app, but it also allows you to share content between multiple apps and extensions that use the same App Group
.
Your suiteName
cannot be the same as your apps bundle identifier
. Using the global domain
is also not allowed as it is not writeable by apps.
Step 1: Create new suite
Creating a new suite
with UserDefaults
is super simple. All we have to do is initialize UserDefaults
with the suiteName
that we want to use.
I am going to create two suites so that we can see later on how each suite has a different value
for the same key
.
I am doing everything in the viewDidLoad
for this step so that I cannot use these instances of UserDefaults
later on.
override func viewDidLoad() {
super.viewDidLoad()
// Create the UserDefaults suites
let testSuite = UserDefaults(suiteName: "testSuite")
let appSuite = UserDefaults(suiteName: "appSuite")
}
Step 2: Add data to the different suites
Now that we have created the two suites we need to add some data to them.
override func viewDidLoad() {
super.viewDidLoad()
// Create the UserDefaults suites
let testSuite = UserDefaults(suiteName: "testSuite")
let appSuite = UserDefaults(suiteName: "appSuite")
// Set values in both suites
testSuite?.set("My test value for defaults",
forKey: "sameKey")
appSuite?.set("My app value for defaults",
forKey: "sameKey")
}
In the above code I updated my viewDidLoad
method to set a unique value for my testSuite
and my appSuite
. Both suites use the same value for their key, in this example the key is sameKey
.
Step 3: Getting the data out of UserDefaults
Now all we need to do is to get the unique data out from each suite
that we created. To do this I am going to create two super simple methods which will be name fromTestSuite
and fromAppSuite
.
The code is as follows:
private func fromTestSuite()
{
let testSuite = UserDefaults(suiteName: "testSuite")
let valueForSameKey = testSuite?.object(forKey: "sameKey")
print("Test Suite: ", valueForSameKey)
}
private func fromAppSuite()
{
let appSuite = UserDefaults(suiteName: "appSuite")
let valueForSameKey = appSuite?.object(forKey: "sameKey")
print("App Suite: ", valueForSameKey)
}
All these methods do is create a new instance for the appropriate suite. It will then get the value for sameKey
and print that out to the console.
Step 4: Calling the above methods
All we have to do now is call the above methods. Once again I have put this code into my viewDidLoad
method as shown below:
override func viewDidLoad() {
super.viewDidLoad()
// Create the UserDefaults suites
let testSuite = UserDefaults(suiteName: "testSuite")
let appSuite = UserDefaults(suiteName: "appSuite")
// Set values in both suites
testSuite?.set("My test value for defaults",
forKey: "sameKey")
appSuite?.set("My app value for defaults",
forKey: "sameKey")
// Call print the values in the console.
self.fromTestSuite()
self.fromAppSuite()
}
If you run the app now you should see the unique values from each suite
using the same key.