Get keyboard height with Swift
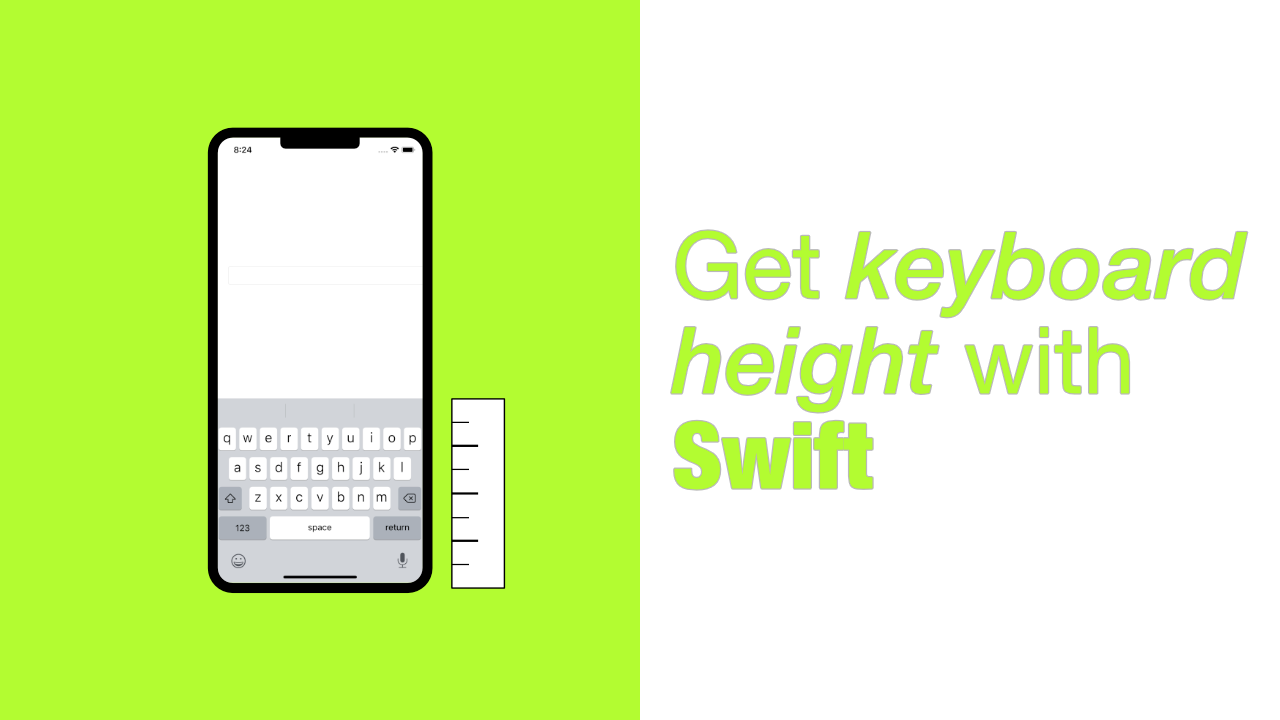
Getting the keyboard height with Swift is easy, and can be used when we need to move some content up if the keyboard overlaps it.
To get the keyboard height we need to listen for the.keyboardDidShowNotification
. When receive this notification we can access the keyboard height from the notification'suserInfo
.
Step 1: Listen for keyboardDidShowNotification
When the keyboard becomes visible or gets hidden it will post a notification. When it becomes visible it will post a notification with this notification name .keyboardDidShowNotification
.
To listen for this notification, add the following notification observer to your code.
NotificationCenter.default.addObserver(self,
selector: #selector(handle(keyboardShowNotification:)),
name: UIResponder.keyboardDidShowNotification,
object: nil)
I have added this into my ViewDidLoad
so that the observer will be added once the view controller has loaded.
In the above code you can see that for my #selector
I am using handle(keyboardShowNotification:)
. You don't have this method yet so let's add it.
Step 2: Get keyboard height
The keyboardDidShowNotification
contains the keyboard height in the notification userInfo
. To get the height from the userInfo
let's create the method that was mentioned in the previous step.
To that that, add the following code to your file:
@objc
private func handle(keyboardShowNotification notification: Notification) {
// 1
print("Keyboard show notification")
// 2
if let userInfo = notification.userInfo,
// 3
let keyboardRectangle = userInfo[UIResponder.keyboardFrameEndUserInfoKey] as? CGRect {
print(keyboardRectangle.height)
}
}
Let's go through this code:
- Simply print
Keyboard show notification
to make sure that this method is being called when the keyboard is shown. - We use an
if let
to ensure thatnotification.userInfo
is notnil
. - If it is not
nil
we will try and get the keyboards frame. To do this we use theUIResponder.keyboardFrameEndUserInfoKey
key. The value for this key is anNSValue
, but we can cast it to aCGRect
immediately. ThisCGRect
will contain the frame of the keyboard.
This is what the Apple documentation says about .keyboardFrameEndUserInfoKey
:
The key for an NSValue object containing a CGRect that identifies the ending frame rectangle of the keyboard in screen coordinates
If this is not nil, keyboardRectangle
will have a value. This value will be a CGRect
because that is what we cast it to. Because it is a CGRect
we can get the height by doing this:
keyboardRectangle.height
When we implemented the handle(keyboardShowNotification notification: Notification)
above, we printed out keyboardRectangle.height
but you can now use it however you need to.
NOTE: Please ensure that you are following best practice when listening for notifications. In this article I am only showing you how to get the height from the notification, but the notifications should be removed when it is appropriate to do so.