Python list all files in directory and subdirectories
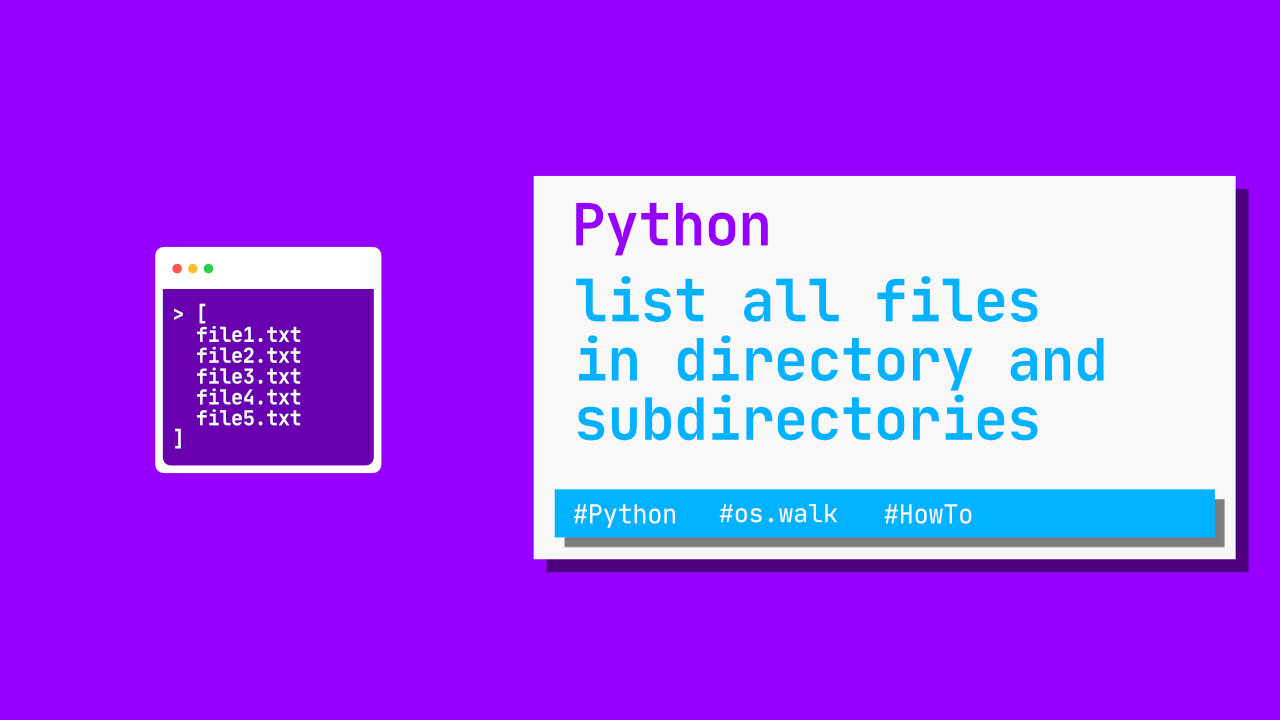
Getting a list of all files in a directory and its subdirectories can be quite a common task, so, in this tutorial I will show you how you can do this with 4 lines of code using os.walk
.
Below you can see how we can recursively loop through all the files in a given directory:
import os
for path, currentDirectory, files in os.walk("/Users/darren/Desktop/test"):
for file in files:
print(os.path.join(path, file))
In the above code I join the path
and the file
to give me the full path to each file that exists within the directory that I provided.
When I run the above code I have the following output:
/Users/darren/Desktop/test/.DS_Store
/Users/darren/Desktop/test/file 1.rtf
/Users/darren/Desktop/test/test 1 level/.DS_Store
/Users/darren/Desktop/test/test 1 level/file 2.rtf
There are a few other ways to do this, but I prefer to use os.walk
because of how simple it is to use.