Python - list all files starting with given string/prefix
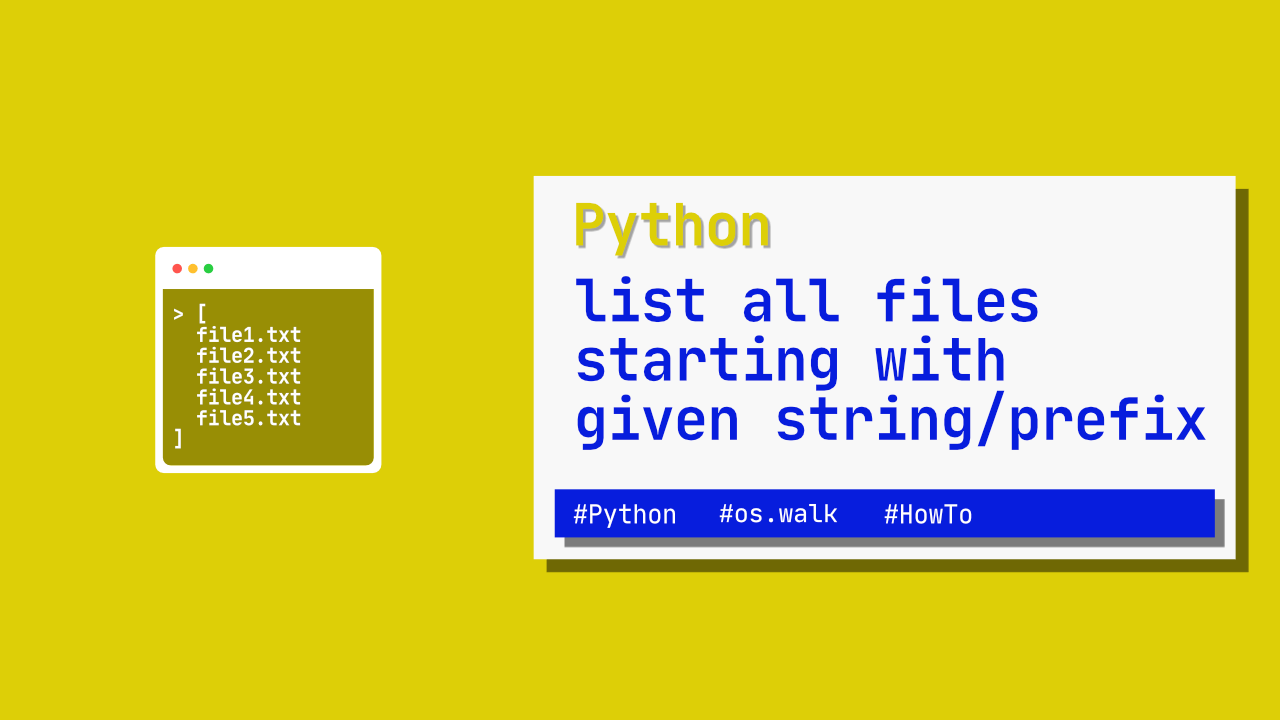
In this tutorial I will show you how to list all files in a directory where those files start with a given string/prefix.
Find files in the current directory
To loop through the provided directory, and not subdirectories we can use the following code:
for file in os.listdir("/Users/darren/Desktop/test"):
if file.startswith("art"):
print(file)
The above code will loop through all the files in my test
directory. On each iteration it will check to see if the filename starts with art
, if it does, it will print it out. In my case, the following prints out:
article 1.rtf
Find files recursively
In the first section we looked at how we can list all files in a given directory with a given string/prefix. In this section we will look at how we can do this recursively, meaning, listing all files in the given directory and all of its subdirectories where the file starts with a given string/prefix.
for path, currentDirectory, files in os.walk("/Users/darren/Desktop/test"):
for file in files:
if file.startswith("art"):
print(file)
The code is very similar, but now we use os.walk
instead of os.listdir
. os.walk
will allow us to go through all the subdirectories as well.
In each directory we loop through each file. We will then check to see if that file's name starts with art
and if it does we will print out the file name. In my case, it prints the following:
article 1.rtf
article 2.rtf
article 3.rtf
If you want to print out the full path for the file you can replace print(file)
with:
print(os.path.join(path, file))
If I run this I get the following output:
/Users/darren/Desktop/test/article 1.rtf
/Users/darren/Desktop/test/test 1 level/article 2.rtf
/Users/darren/Desktop/test/test 1 level/test 2 level/article 3.rtf