Remove empty rows/cells in table view
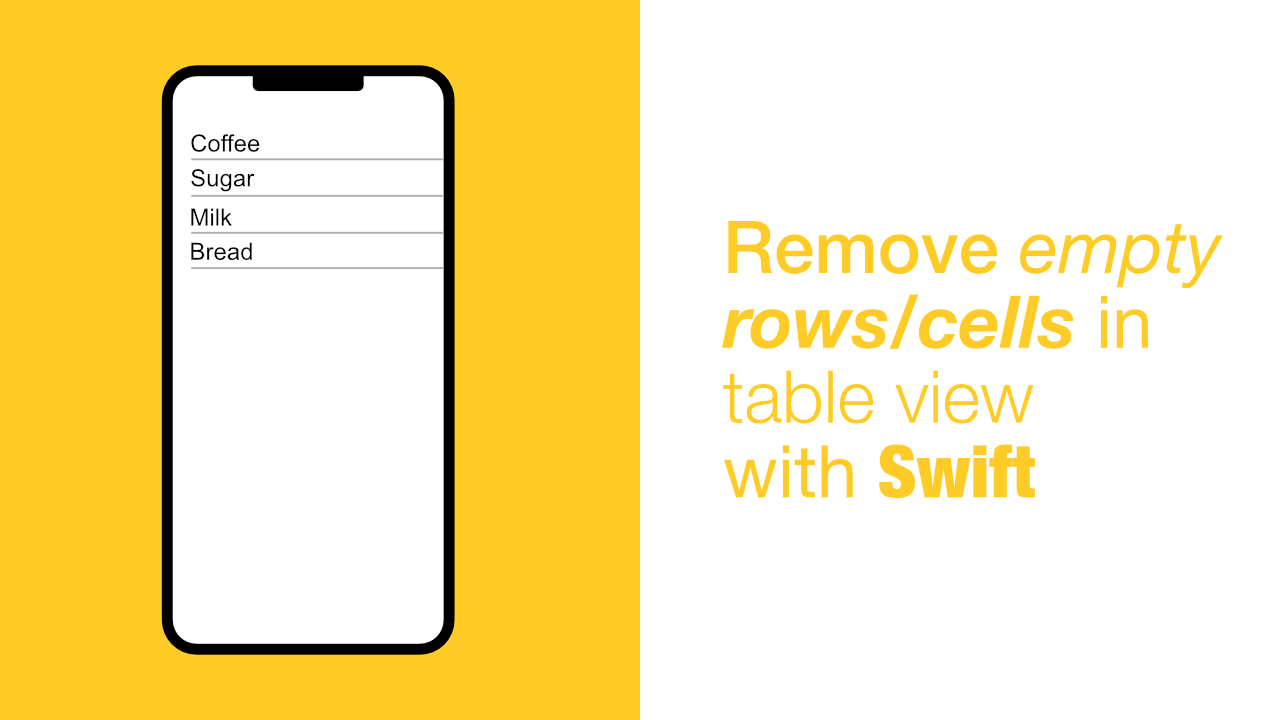
When a UITableView
only has a few element the empty rows/cells will still be visible. In this tutorial we will learn how to remove the empty rows, and to remove the separator from the empty rows is quite simple.
To remove empty rows in a table view, all you need to do is to add a footer view to the table view. We will look at how this is done later in the tutorial
This is the issue that we want to fix:
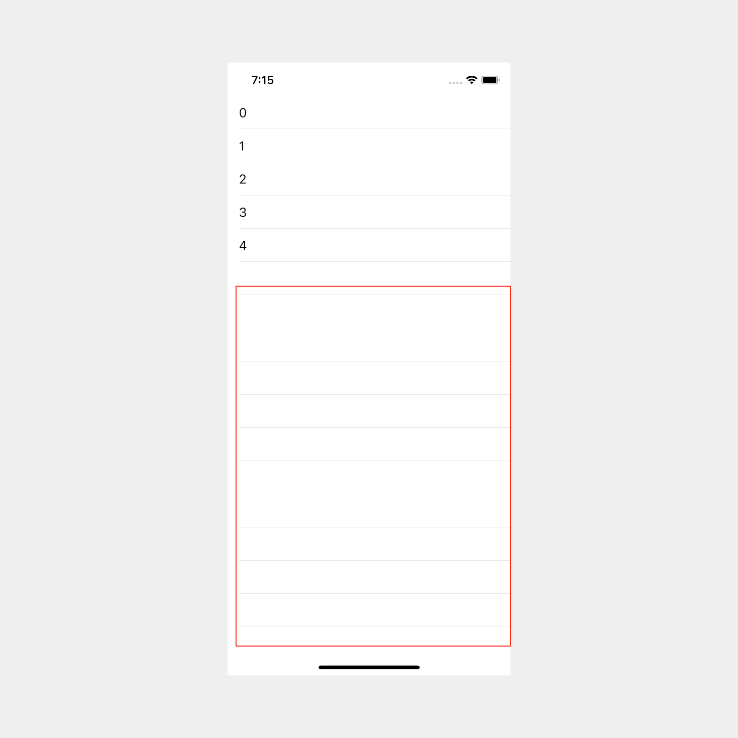
If you have custom cells you could simply set separator style to .none
, like this:
self.tableView.separatorStyle = .none
Setting the .separatorStyle
to .none
will remove all the separators, which is not what we want and it will look like this:
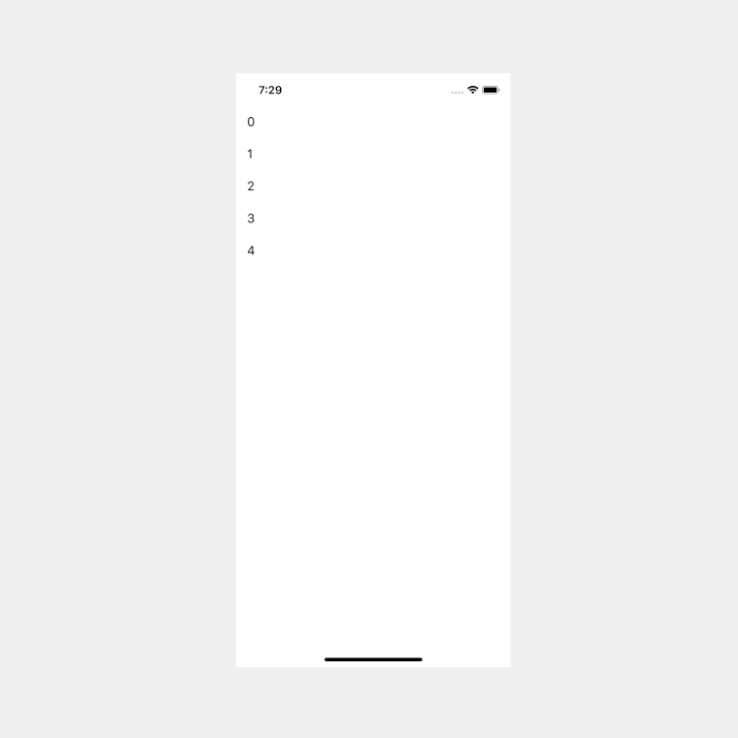
If you are using custom table view cells you would be able to set
to separatorStyle
.none
. When I use custom cells I will always remove the default separator and create my own separator in the cell itself, that way I have full control over it.
Using a custom cell can therefore be a solution to this issue, but it might be overkill if you have a simple cell.
The best way to do this is to set a footer view to the table view. There are two ways to do this. You can do it with code, or you can do it with the Interface Builder. We will go through both ways.
Add footer view with code
Adding a footer with code is the easier option in my opinion. UITableView
has a built in method that will allow us to easily add a footer view.
To add a footer view with code, add the following code to your project:
func tableView(_ tableView: UITableView,
viewForFooterInSection section: Int) -> UIView? {
return UIView()
}
Add footer view with Interface Builder
To add a footer with Interface Builder, all you need to do is drag a new view underneath the prototype cells. See below:
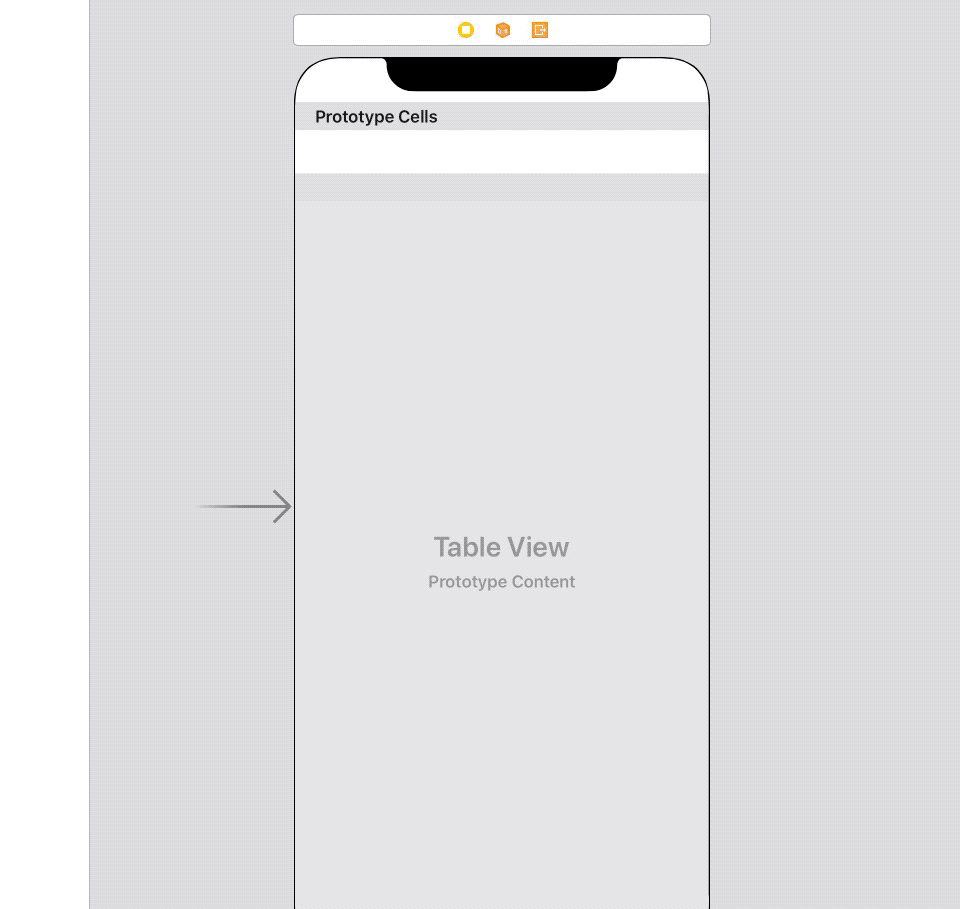
If you build and run your app after either of these solutions, the tableview should look like this:
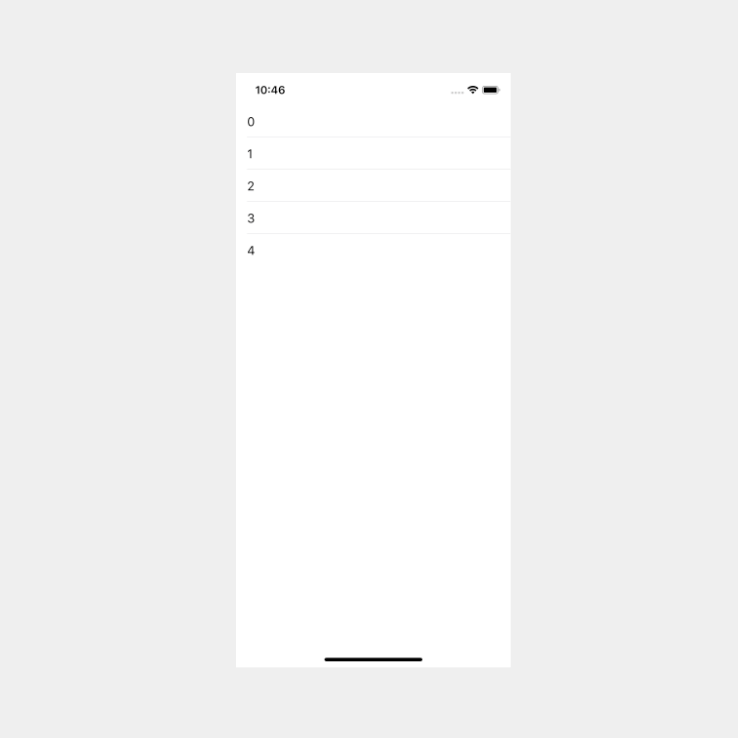
Conclusion
Removing the empty rows is quite simple, but it might not be how you expected it to be. Not that you know how to do it, it will be extremely easy next time.
If you want to find the full source code for this tutorial, it can be found here.