Remove UITableViewCell highlight/selection color with Swift
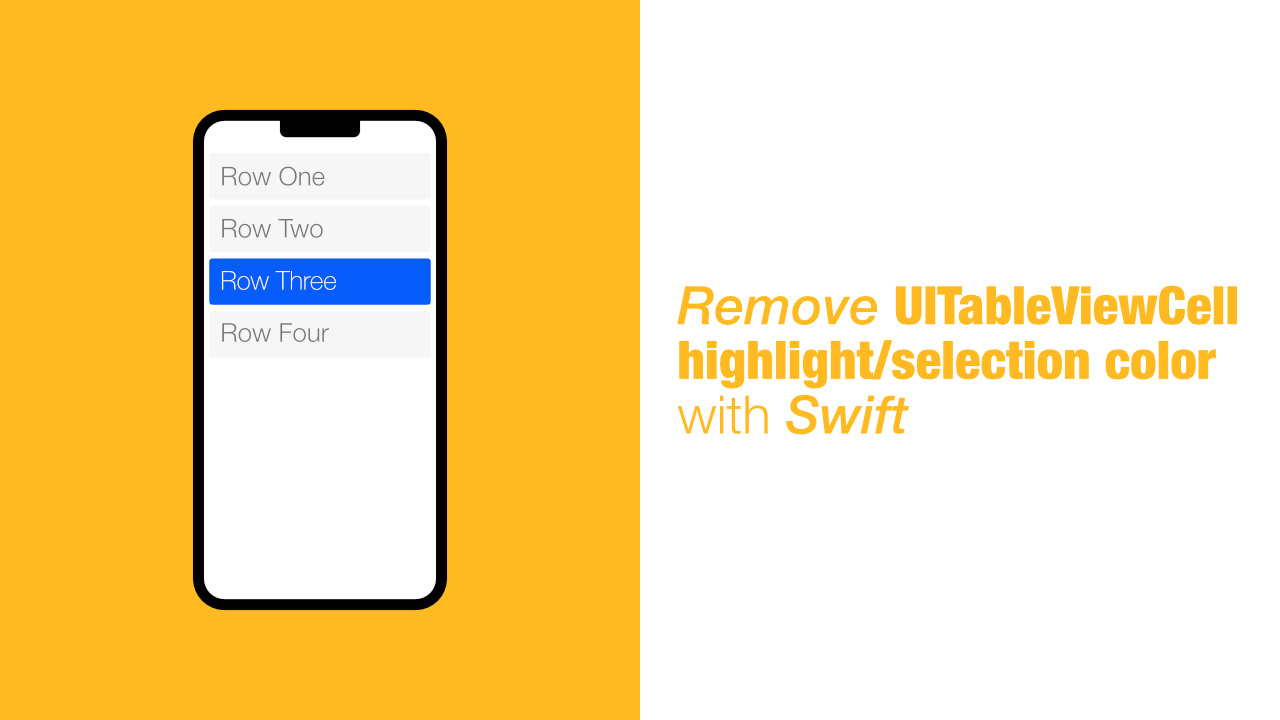
This is one of those annoying default behaviours that iOS has. Below is a one liner that will help you remove the cell highlighting.
To remove the table view cell highlight/selection color all you need to do is this:
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = UITableViewCell()
cell.textLabel?.text = "My cell text"
// Remove cell highlight/selection color
cell.selectionStyle = .none
return cell
}
Inside the cellForRowAt indexPath
method, on the table view cell, set the selectionStyle
to .none
.
Using an Extension
It is as easy as that to remove the highlight/selection color from a UITableViewCell. One could make a very simple extension method that can make this a bit easier in terms of autocomplete:
extension UITableViewCell {
func noSelectionStyle() {
self.selectionStyle = .none
}
}
Instead of using the .selectionStyle
property directly, we can now just call this function, so the updated code would look like this:
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = UITableViewCell()
cell.textLabel?.text = "My cell text"
// Remove cell highlight/selection color
cell.noSelectionStyle()
return cell
}
Using a Protocol and Extension
Another way to do this would be to create a subclass of UITableViewCell and have that cell automatically set the selection style to .none
. If you are going to have a subclass, it probably means that you have custom cells, in that case, it would be better to create a protocol that all the cells that require this functionality need to adopt, and have an extension that implements the protocol.
That protocol and extension can look something like this:
protocol NonHighlightableCell {
func noSelectionStyle()
}
extension UITableViewCell: NonHighlightableCell {
func noSelectionStyle() {
self.selectionStyle = .none
}
}
We are using the same extension function here, but now it will only work for cells that adopt the NonHighlightableCell
protocol.